Python Turtle Graphics Tutorial
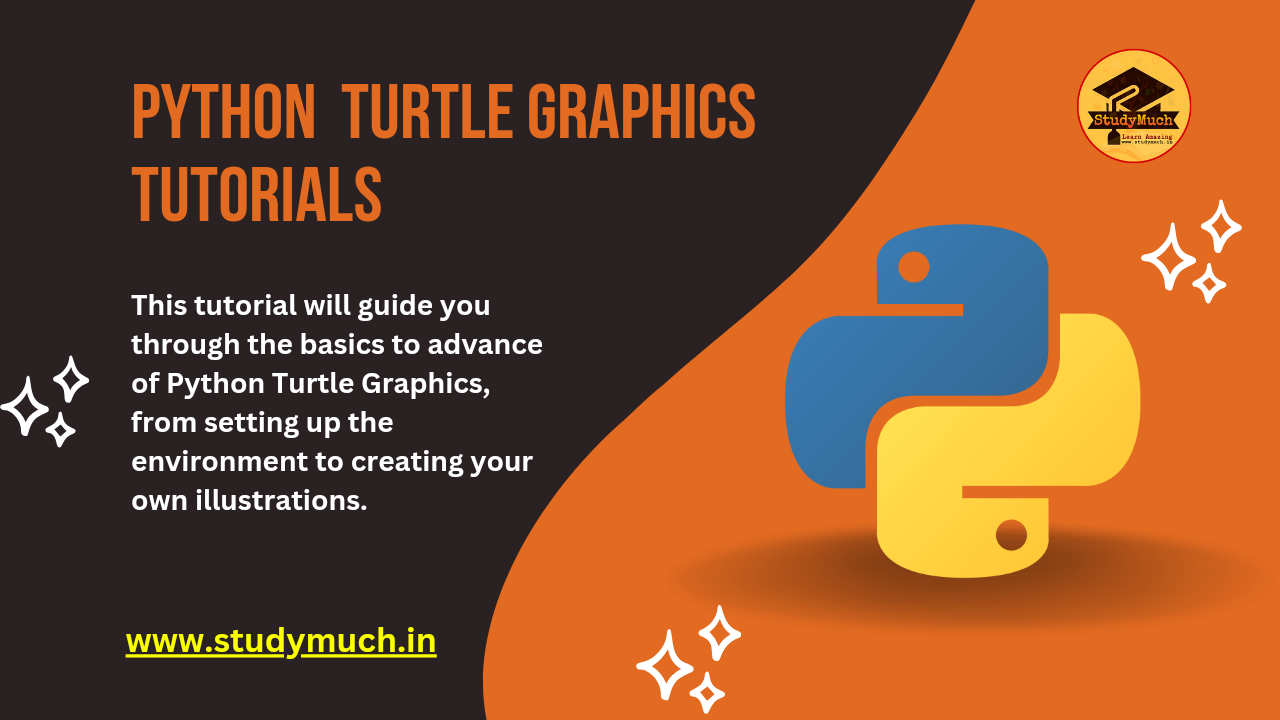
Python Turtle Graphics Tutorial
Python Turtle Graphics is an essential and fun way to get introduced to programming and computer graphics. The Turtle module in Python is a standard way of creating graphics programs that allows you to create shapes, patterns, and designs using a virtual “turtle”. This tutorial will guide you through the basics to advance of Python Turtle Graphics, from setting up the environment to creating your own illustrations.
Getting Started
Installing Python and Turtle
Before we start, ensure you have Python installed on your computer. You can download it from the official Python Website. The Turtle module is included in the Python standard library, so you don’t need to install it separately.
Setting Up Your Environment
You can use any code editor to write your Turtle programs, such as IDLE (which comes with Python), VS Code, PyCharm, or any other editor of your choice.
Basic Concepts
Importing the Turtle Module
To use Turtle, you need to import the module at the beginning of your program:
import turtle
Creating a Turtle Object
Next, create a Turtle object, which you will use to control the turtle:
import turtle
my_turtle = turtle.Turtle()
Moving the Turtle
You can move the turtle forward, backward, and turn it left or right using the following methods:
import turtle
my_turtle = turtle.Turtle()
my_turtle.forward(100) # Move forward by 100 units
my_turtle.backward(50) # Move backward by 50 units
my_turtle.right(90) # Turn right by 90 degrees
my_turtle.left(45) # Turn left by 45 degrees
Drawing Shapes
You can use the turtle to draw various shapes. Here are some examples:
Drawing Square:
import turtle
my_turtle = turtle.Turtle()
for _ in range(4):
my_turtle.forward(100)
my_turtle.right(90)
Drawing Triangle:
import turtle
my_turtle = turtle.Turtle()
for _ in range(3):
my_turtle.forward(100)
my_turtle.right(120)
Drawing Circle:
mport turtle my_turtle = turtle.Turtle() my_turtle.circle(50) # Draw a circle with radius 50
Customizing the Turtle
You can customize the appearance of the turtle and the drawing. Here are some options:
Changing the Turtle’s Shape
You can change the shape of the turtle:
import turtle
my_turtle = turtle.Turtle()
my_turtle.shape("turtle") # Other options include "arrow", "circle", etc.
my_turtle.circle(50) # Draw a circle with radius 50
Changing the Pen Color and Size
You can change the color and size of the pen:
import turtle
my_turtle = turtle.Turtle()
my_turtle.pencolor("red") # Set the pen color to blue
my_turtle.pensize(5) # Set the pen size to 5
my_turtle.shape("turtle") # Other options include "arrow", "circle", "square", etc.
my_turtle.circle(50) # Draw a circle with radius 50
Filling Shapes with Color
You can fill shapes with color using the begin_fill and end_fill methods:
import turtle
my_turtle = turtle.Turtle()
my_turtle.pencolor("red") # Set the pen color to blue
my_turtle.pensize(5) # Set the pen size to 5
my_turtle.fillcolor("red") # Set the fill color to red
my_turtle.begin_fill()
my_turtle.shape("turtle") # Other options include "arrow", "circle", "square", etc.
my_turtle.circle(50) # Draw a circle with radius 50
my_turtle.end_fill()
Conclusion
Python Turtle is a great way to learn graphics programming and create visually appealing designs. By mastering the basics and experimenting with advanced techniques, you can create complex and beautiful graphics. Have fun exploring the world of Turtle Graphics and see where your creativity takes you!
Learn More;
0 Comments