Advance Turtle Graphics Tutorial
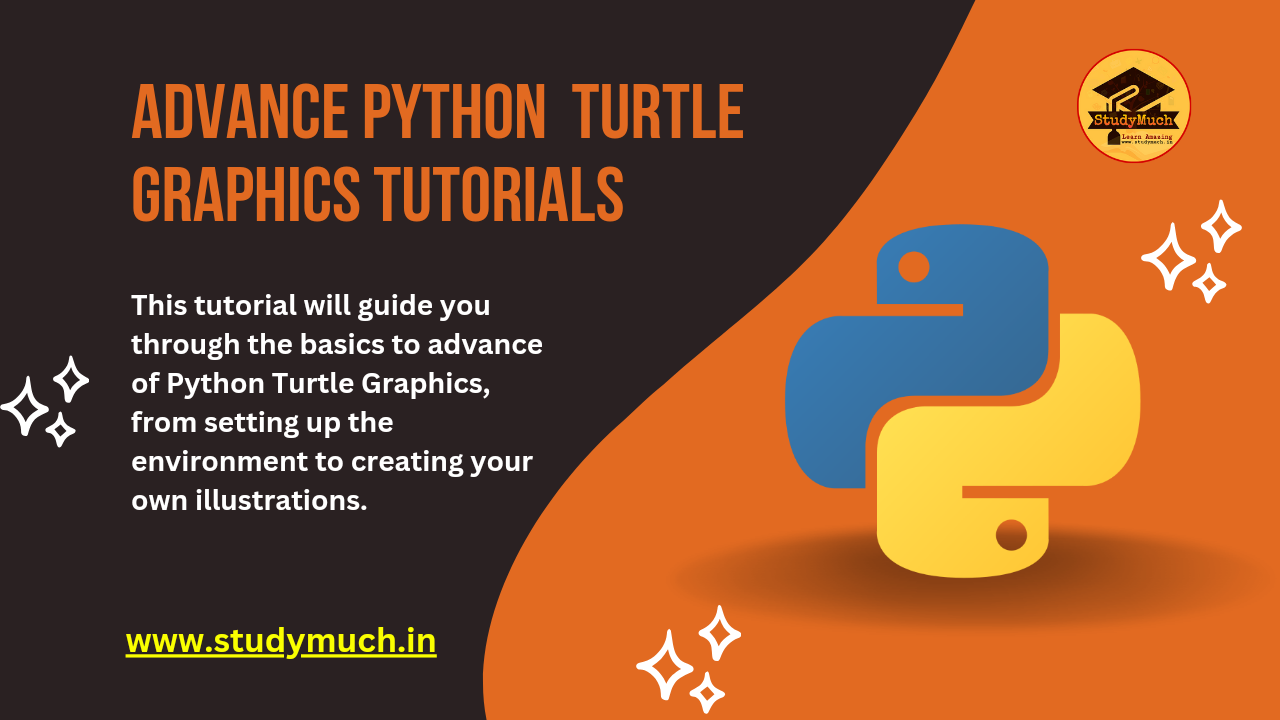
Advance Turtle Graphics Tutorial:
In this advanced Python Turtle Graphics tutorial, you will learn how to create complex patterns and different designs. This guide will help you explore more sophisticated techniques and unleash your creativity using Python’s Turtle module.
Creating Patterns
You can create intricate patterns using loops and Turtle commands. Here is an example of a star pattern:
import turtle
my_turtle = turtle.Turtle()
my_turtle.pencolor("red") # Set the pen color to blue
my_turtle.pensize(5) # Set the pen size to 5
my_turtle.shape("turtle") # Other options include "arrow", "circle", "square", etc.
for _ in range(18):
my_turtle.forward(200)
my_turtle.right(170)
Animations
You can animate your turtle graphics by controlling the drawing speed and using loops:
import turtle
my_turtle = turtle.Turtle()
my_turtle.pencolor("red") # Set the pen color to blue
my_turtle.pensize(3) # Set the pen size to 5
my_turtle.speed(1) # Slow speed
for _ in range(36):
my_turtle.forward(100)
my_turtle.right(170)
my_turtle.speed(10) # Fast speed for the next iteration
Handling Events
You can handle user events like key presses and mouse clicks to make your Turtle graphics interactive:
import turtle
my_turtle = turtle.Turtle()
def move_forward():
my_turtle.forward(100)
turtle.listen() # Set the window to listen for events
turtle.onkey(move_forward, "Up") # Call move_forward when the Up arrow key is pressed
my_turtle.done
Creating Your Own Designs
Now that you have the basics, you can create your own designs and drawings. Experiment with different shapes, patterns, and colors to see what you can create.
To Saving Your Work
You can save your Turtle graphics as an image file. To do this, you need to use the Screen object:
screen = turtle.Screen()
screen.getcanvas().postscript(file="turtle_drawing.eps")
To Keep the Window Open:
The turtle.done() function is called to keep the Turtle graphics window open until the user closes it.
# Keep the window open
turtle.done()
Drawing Star Patterns:
Star patterns are a great way to understand loops and angles. Here’s how to draw a simple star:
import turtle
my_turtle = turtle.Turtle()
def draw_star(size):
for _ in range(5):
my_turtle.forward(size)
my_turtle.right(144)
# Draw a star with a side length of 100 units
draw_star(100)
Drawing Spirograph:
A spirograph is a complex pattern that looks beautiful and is fun to create. It involves drawing circles in a loop with slight rotations.
import turtle
my_turtle = turtle.Turtle()
my_turtle.speed(0) # Fastest speed
my_turtle.pensize(3)
def draw_spirograph(radius, angle):
for _ in range(int(360 / angle)):
my_turtle.circle(radius)
my_turtle.right(angle)
# Draw a spirograph with a radius of 100 units and an angle of 10 degrees
draw_spirograph(100, 10)
turtle.done
Drawing Fractal Trees;
Fractal trees are a popular pattern in computer graphics, demonstrating recursion and geometric beauty.
import turtle my_turtle = turtle.Turtle() my_turtle.pensize(3) my_turtle.speed(0) # Fastest speed def draw_branch(branch_length): if branch_length > 5: my_turtle.forward(branch_length) my_turtle.right(20) draw_branch(branch_length - 15) my_turtle.left(40) draw_branch(branch_length - 15) my_turtle.right(20) my_turtle.backward(branch_length) # Set the initial position and orientation my_turtle.left(90) my_turtle.up() my_turtle.backward(100) my_turtle.down() my_turtle.color("green") # Draw a fractal tree draw_branch(100)
Mandala Patterns
Mandalas are intricate circular designs that can be created using loops and rotations.
import turtle my_turtle = turtle.Turtle() my_turtle.pensize(3) my_turtle.pencolor("green") my_turtle.speed(0) # Fastest speed def draw_mandala(size, layers): for _ in range(layers): for _ in range(6): my_turtle.forward(size) my_turtle.right(60) my_turtle.right(360 / layers) # Draw a mandala with a size of 50 units and 36 layers draw_mandala(50, 36)
Customizing Patterns
Customizing your Turtle graphics patterns can make your drawings more vibrant and unique. By changing the pen color, fill color, and pen size, you can enhance the visual appeal of your designs. You can further customize these patterns by changing the pen color, fill color, and pen size.
my_turtle.pencolor("blue")
my_turtle.fillcolor("cyan")
my_turtle.pensize(2)
Conclusion
Advanced Python Turtle Graphics allows you to create stunning patterns and designs by taking advantage of loops, recursion, and event handling. By experimenting with different shapes, colors and patterns, you can create complex and beautiful graphics. Happy coding and enjoy exploring the infinite possibilities with Python Turtle Graphics! (Advance Turtle Graphics Tutorial).
Learn More;
2 Comments
Free URL Shortener · September 2, 2024 at 7:26 pm
Hey, I’m Jack. Your blog is a game-changer! The content is insightful, well-researched, and always relevant. Great job!
smortergiremal · November 4, 2024 at 9:04 am
Nice post. I learn something more challenging on different blogs everyday. It will always be stimulating to read content from other writers and practice a little something from their store. I’d prefer to use some with the content on my blog whether you don’t mind. Natually I’ll give you a link on your web blog. Thanks for sharing.