Python Assignment Operators
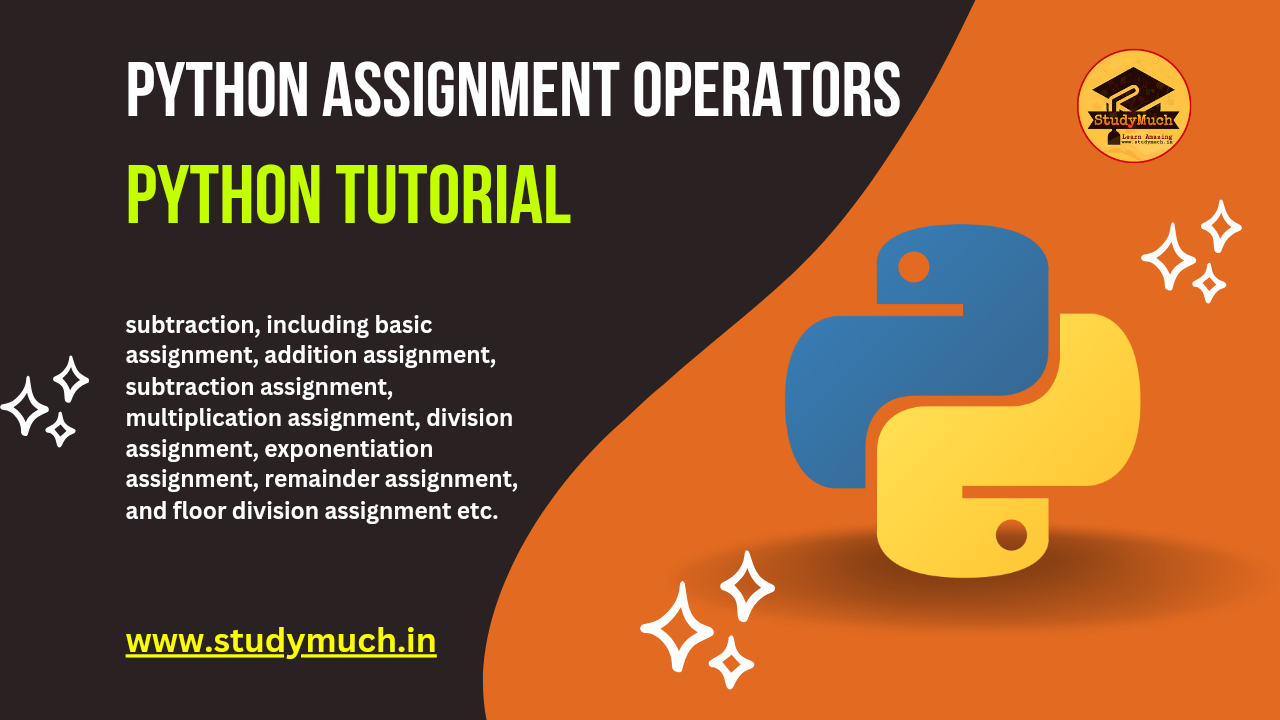
Python Assignment Operators: A Comprehensive Guide
In Python, assignment operators are an important part of the language’s syntax. They allow you to assign values to variables while performing different operations in the same step. In this blog post, we will learn about Python Assignment Operators in detail, including basic assignment, addition assignment, subtraction assignment, multiplication assignment, division assignment, exponentiation assignment, remainder assignment, and floor division assignment.
Here is a list of Python Assignment Operator;
Operator | Example | Description |
= | x = 3 | Assignment |
+= | x += 3 | Addition Assignment |
-= | x -= 3 | Subtraction Assignment |
*= | x *= 3 | Multiplication Assignment |
/= | x /= 3 | Division Assignment |
**= | x **= 3 | Exponentiation Assignment |
%= | x %= 3 | Remainder Assignment |
//= | x //= 3 | Floor Division Assignment |
Basic Assignment (=)
The basic assignment operator (=) is used to assign a value to a variable.
s = "StudyMuch" print(s) #Output - StudyMuch
Addition Assignment (+=)
The addition assignment operator (+=) adds the right operand to the left operand and assigns the result to the left operand.
y = 3 y += 2 print(y) #Output - 5
In this example, the value of y is incremented by 2, and the result (5) is stored back in y.
Subtraction Assignment (-=)
The subtraction assignment operator (-=) subtracts the right operand from the left operand and assigns the result to the left operand.
a = 10 a -= 4 print(a) #Output - 6
Here, the value of a is reduced by 4, resulting in a being assigned the value 6.
Multiplication Assignment (*=)
The multiplication assignment operator (*=) multiplies the left operand by the right operand and assigns the result to the left operand.
b = 7 b *= 3 print(b) #Output- 21
In this case, the value of b is multiplied by 3, making b equal to 21.
Division Assignment (/=)
The division assignment operator (/=) divides the left operand by the right operand and assigns the result to the left operand. It always results in a floating-point number.
c = 10 c /= 2 print(c) #Output- 5.0
The value of c is divided by 2, and c is assigned the value 5.0.
Exponentiation Assignment (**=)
The exponentiation assignment operator (**=) raises the left operand to the power of the right operand and assigns the result to the left operand.
d = 2 d **= 3 print(d) #Output- 8
In this example, d is raised to the power of 3, and d becomes 8.
Remainder Assignment (%=)
The remainder assignment operator (%=) calculates the remainder when the left operand is divided by the right operand and assigns the result to the left operand.
e = 11 e %= 3 print(e) #Output-2
The remainder when 11 is divided by 3 is 2, so e is assigned the value 2.
Floor Division Assignment (//=)
The floor division assignment operator (//=) divides the left operand by the right operand, rounds down to the nearest integer, and assigns the result to the left operand.
f = 10 f //= 3 print(f) #Output-3
The floor division of 10 by 3 is 3, and f is assigned this value.
Conclusion
Python’s assignment operators provide a concise and efficient way to perform operations while assigning values to variables. Whether you need to add, subtract, multiply, divide, exponentiate, find the remainder, or perform floor division, these operators can simplify your code and make it more readable. Understanding how assignment operators work is essential for effective Python programming. I hope you have understood this tutorial better, but you have any doubt then you can ask in the comment section.
Learn More;
- Learn full Tutorial of Python.
- Learn Tutorial of JavaScript.
- Learn Tutorial of HTML and CSS.
- Learn Future of Artificial Intelligence.
0 Comments