Python Membership Operators
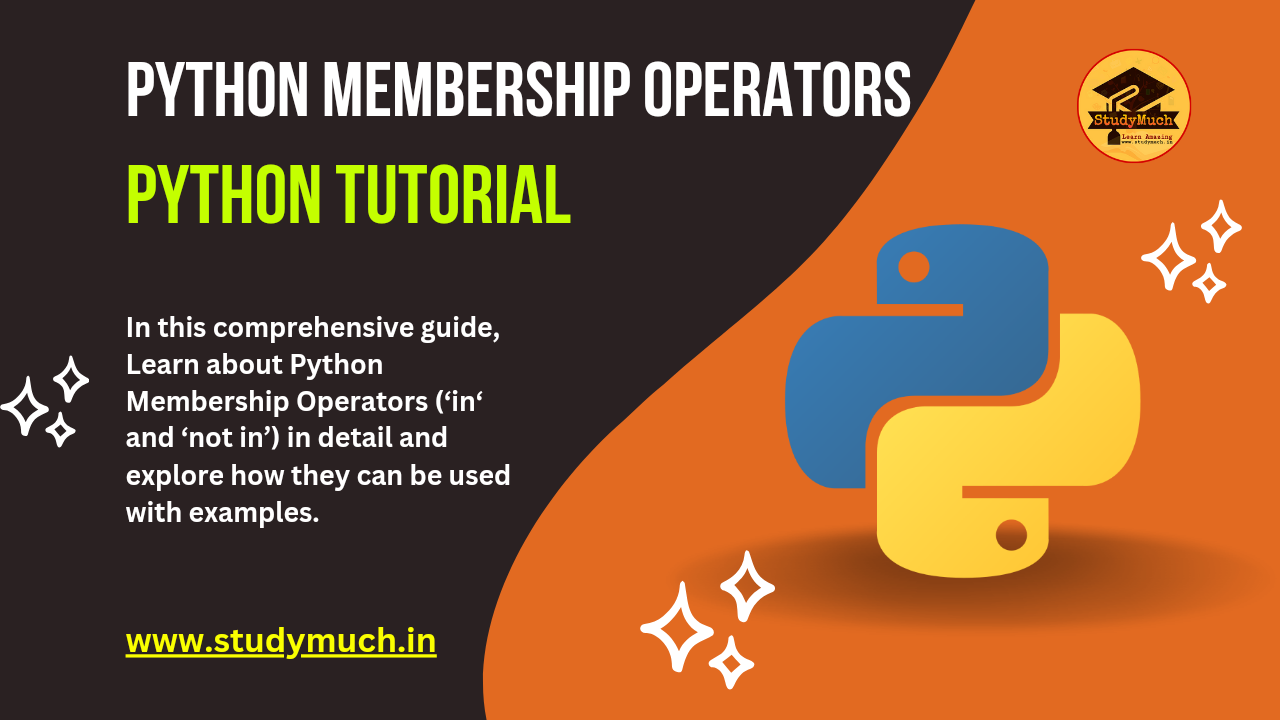
Python Membership Operators: Explained with Examples
Python provides a series of operators to perform various operations on data. Of these, membership operators are particularly useful for working with sequences such as strings, lists, and tuples. In this blog post, we will learn about Python Membership Operators (‘in‘ and ‘not in’) in detail and explore how they can be used with examples.
What are Membership Operators?
Membership operators are used to test whether a value is a member of a sequence.
There are two membership operators in Python:
- ‘in‘: Returns ‘True’ if a value is found in the sequence.
- ‘not in’: Returns ‘True’ if a value is not found in the sequence.
These operators are often employed in conditional statements to make decisions based on whether a value exists within a collection.
Using the ‘in’ Operator
The ‘in’ operator checks if a given value exists in a sequence. It returns ‘True’ if the value is found and ‘False’ otherwise.
Let’s look at some examples:
# Check if an element exists in a list list = [1, 2, 3, 4, 5] print(3 in list) # True
In the above example, 3 is present in list, so result will be True.
# Check if a character exists in a string str = "Hello, World!" print('o' in str) # True
In this case, the character ‘o’ is present in str, so result is True.
Using the ‘not in’ Operator
The ‘not in’ operator, as the name suggests, checks if a value is not present in a sequence. It returns True if the value is absent and False if it is found.
Here are some examples:
# Check if an element does not exist in a list list = [1, 2, 3, 4, 5] print (6 not in list) # True
In this example, 6 is not present in ‘list’, so result is ‘True’.
# Check if a substring is not in a string str = "Hello, World!" print('Python' not in str) # True
Since ‘Python’ is not found in ‘str’, result is ‘True’.
Using Membership Operators in Conditional Statements
Membership operators are often used in if statements to conditionally execute code based on whether a value is present in a sequence or not. Here’s an example:
fruits = ['apple', 'banana', 'cherry'] fruit = input("Enter a fruit: ") if fruit in fruits: print(f"{fruit} is in the list of fruits.") else: print(f"{fruit} is not in the list of fruits.")
In the above example, the user is asked to enter a fruit. The if statement checks whether the entered fruit is in the ‘fruits list’ or not. If fruit is in list, it prints a message saying it is in the list; Otherwise, it prints that it is not.
Conclusion;
Python membership operators (‘in’ and ‘not in’) are powerful tools for checking the existence of values within sequences. These operators are particularly handy when working with conditional statements and allow you to make decisions based on whether a value is a member of a collection or not. Incorporate them into your Python code to efficiently handle data and make your programs more robust and user-friendly. I hope you have understood this tutorial better, but if you have any doubts then ask in the comment section.
Learn More;
0 Comments