Drawing a Heart with Python
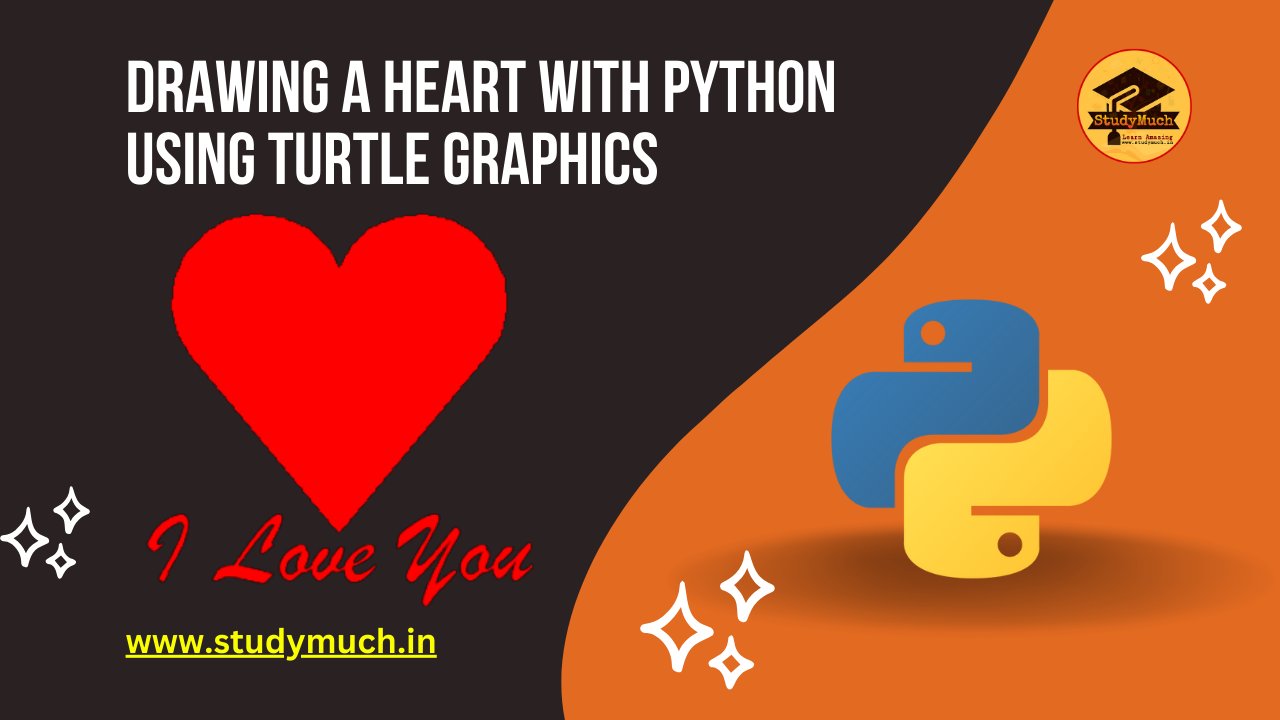
Drawing a Heart with Python Using Turtle Graphics
Python’s Turtle graphics module allows you to create fascinating drawings and patterns using simple commands. In this tutorial, we’ll learn how to draw a Heart shape and add a loving message using Python’s Turtle graphics library. By the end of this guide, you’ll have a beautiful heart-shaped drawing on your screen. (Drawing a Heart with Python)
Prerequisites
Before we start, make sure you have Python and the Turtle graphics library installed on your computer. If not, you can easily install Python from python.org, and Turtle graphics usually comes bundled with Python.
Drawing a Heart Shape
Let’s take a deeper look at the code. You can copy and paste this Python script into your code editor, or follow along step by step:
from turtle import * # Set background color and pen color bgcolor("black") color("red") # Set the title of the drawing window title("StudyMuch") # Begin filling the shape begin_fill() # Customize the pen size pensize(3) # Move the turtle into position left(50) forward(133) circle(50, 200) right(140) circle(50, 200) forward(133) # End the fill end_fill()
Adding a Loving Message
Now, let’s add a loving message to our heart-shaped drawing. We’ll use the penup(), goto(), pendown(), and write()
functions for this:
# Move to a new position penup() goto(0, -50) pendown() # Set the text color and style color("red") write("I Love You", align="center", font=("Brush Script MT", 45, "normal")) # Hide the turtle hideturtle() # Finish the drawing done()
Explanation of Code.
Let’s describe the code;
- We start by setting the background color to black and the pen color to red to give our drawing a vibrant look.
- The title()
function sets the title of the drawing window to “StudyMuch.”
- We begin filling the shape using begin_fill()
and customize the pen size with pensize(3).
- The turtle moves into position to draw the heart shape. We use various turtle commands like left(), forward(), circle(), and right()
to create the heart.
- After finishing the heart shape, we end the fill with end_fill().
- To add the loving message, we use penup()
to lift the pen, goto()
to position the turtle, pendown()
to lower the pen again, and write()
to add the text. We customize the text color and font to make it look lovely.
- Finally, we hide the turtle using hideturtle()
and finish the drawing with done().
Conclusion
Congratulations! You’ve just learned how to create a beautiful heart-shaped drawing with Python’s Turtle graphics library. You can further customize the colors, fonts, and sizes to create your own unique artwork. Turtle graphics is a fun and creative way to explore the world of programming and art. Have fun experimenting and expressing yourself through code! (Drawing a Heart with Python)
Look More;
- Draw BHARAT Flag with Python.
- Draw Flower design with Python.
- Draw Rangoli Design with Python.
- Learn full Tutorial of Python.
0 Comments