Comments in C Programming
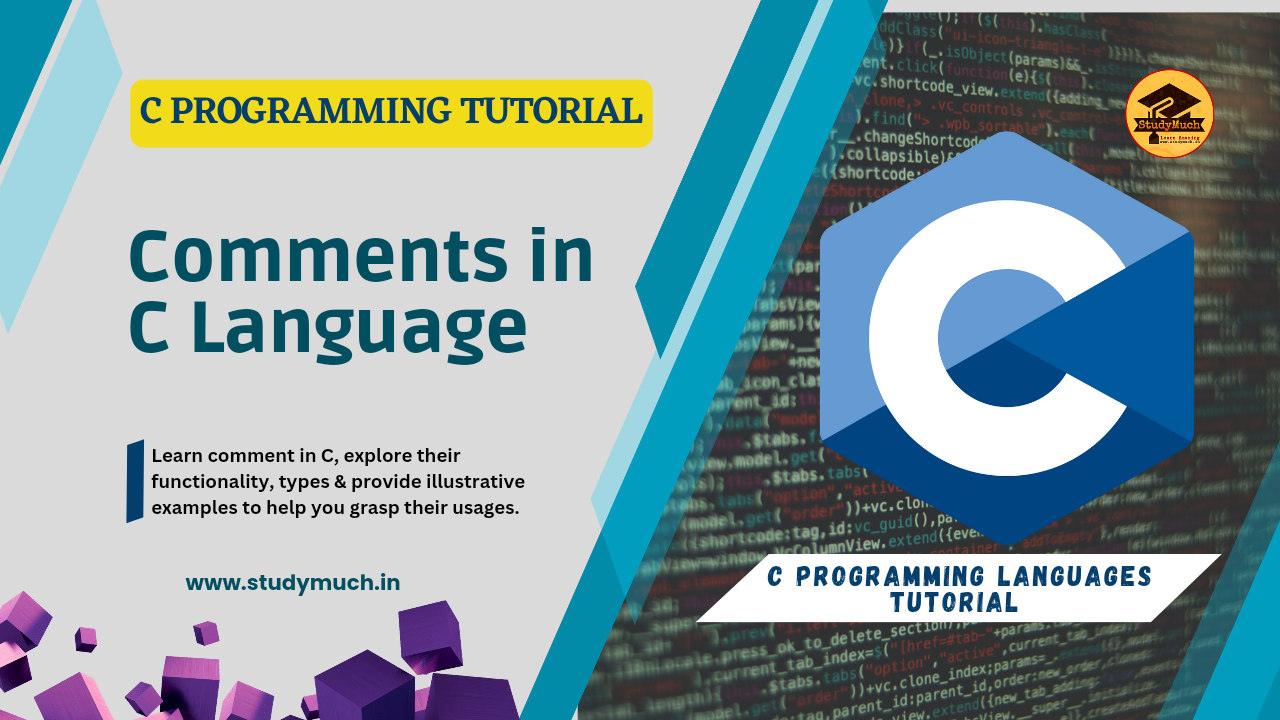
Comments in C Programming
Introduction:
In the world of programming, comments are an invaluable tool that can greatly enhance the clarity, maintainability, and collaboration of code. Comments provide programmers with the ability to add explanatory notes and documentation within their programs, making it easier for others (and even themselves) to understand the code’s purpose and functionality. In this blog post, we will delve into the significance of comments in C programming and explore how they contribute to writing high-quality code.
Types of Comment in C
In C programming, there are two types of comments: single-line comments and multi-line comments.
1. Single-Line Comments:
Single-line comments are used to add explanatory notes or documentation to a specific line of code. They are denoted by two forward slashes “//” at the beginning of the line. Single-line comments extend only to the end of the line and do not continue to subsequent lines. These comments are typically used for brief explanations, reminders, or clarifications. They are often used for:
int age = 25; // Variable to store the age of the user
2. Multi-Line Comments:
Multi-line comments, also known as block comments, are used to provide more extensive documentation or explanations that span multiple lines. They are enclosed within “/” and “/” delimiters. Multi-line comments are useful for describing the purpose of a block of code, documenting algorithms, providing an overview of a program’s structure, or adding copyright information. These comments are often used for:
/* This function calculates the factorial of a given number. It uses a recursive approach to find the factorial. */ int factorial(int num) { if (num == 0 || num == 1) { return 1; } else { return num * factorial(num - 1); } }
Understanding C Programming Comments:
In C programming, comments are textual annotations that are added to the source code and are not executed by the compiler. They serve as informative messages to both programmers and readers of the code. Comments can be written in two forms:
- Single-line comments: Denoted by “//” at the beginning of a line, these comments span only a single line and are often used for brief explanations or reminders.
- Multi-line comments: Enclosed within “/” and “/”, these comments can span multiple lines and are useful for providing more extensive documentation.
Improving Code Readability and Clarity:
One of the primary benefits of using comments in C programming is improving code readability. Well-placed comments can explain the purpose of a code snippet, describe algorithms, provide insight into complex sections, or outline the overall structure of the program. By making the code more understandable, comments enable programmers to maintain, debug, and modify the codebase more efficiently, saving time and effort.
Documenting Code Functionality:
Comments play a crucial role in documenting code functionality. When multiple programmers collaborate on a project, clear and concise comments become essential for understanding each other’s code. Comments can describe the input and output of functions, document assumptions and constraints, and provide usage examples. By documenting code effectively, developers can communicate intentions, making it easier for others to work with the codebase.
Enabling Code Maintenance and Debugging:
Over time, software projects evolve, and codebases grow larger and more complex. In such scenarios, comments become indispensable for code maintenance and debugging. By adding comments to existing code, programmers can leave hints and reminders about potential improvements, known issues, or future considerations. Additionally, during the debugging process, comments can help narrow down the scope of the problem or highlight specific sections that require attention.
Best Practices for Commenting in C Programming:
To ensure effective use of comments, it is essential to follow some best practices:
- Be concise and clear: Write comments that are brief yet informative. Avoid unnecessary verbosity or duplicating code explanations.
- Use proper grammar and punctuation: Comments are part of the code’s documentation, so maintaining good grammar and punctuation is crucial.
- Update comments regularly: As the code evolves, make sure to update or remove outdated comments to avoid confusion.
- Avoid excessive commenting: While comments are valuable, avoid over-commenting code that is self-explanatory. Let the code speak for itself when possible.
Conclusion:
In the realm of C programming, comments serve as a powerful tool for enhancing code clarity, readability, and collaboration. By providing descriptive explanations, comments enable programmers to understand the codebase better, collaborate effectively, and ensure smoother code maintenance and debugging. Adopting best practices for commenting can significantly improve the quality of the code and the productivity of the development team. So, let us embrace the power of comments and make our C programs more accessible and maintainable for ourselves and fellow developers.
Learn More;
1 Comment
Ernestbok · November 10, 2023 at 8:28 pm
Amazing tons of terrific information.
essay writing structure [url=https://topswritingservices.com/#]websites that write essays for you[/url] law essay writing service australia