Data Type in C
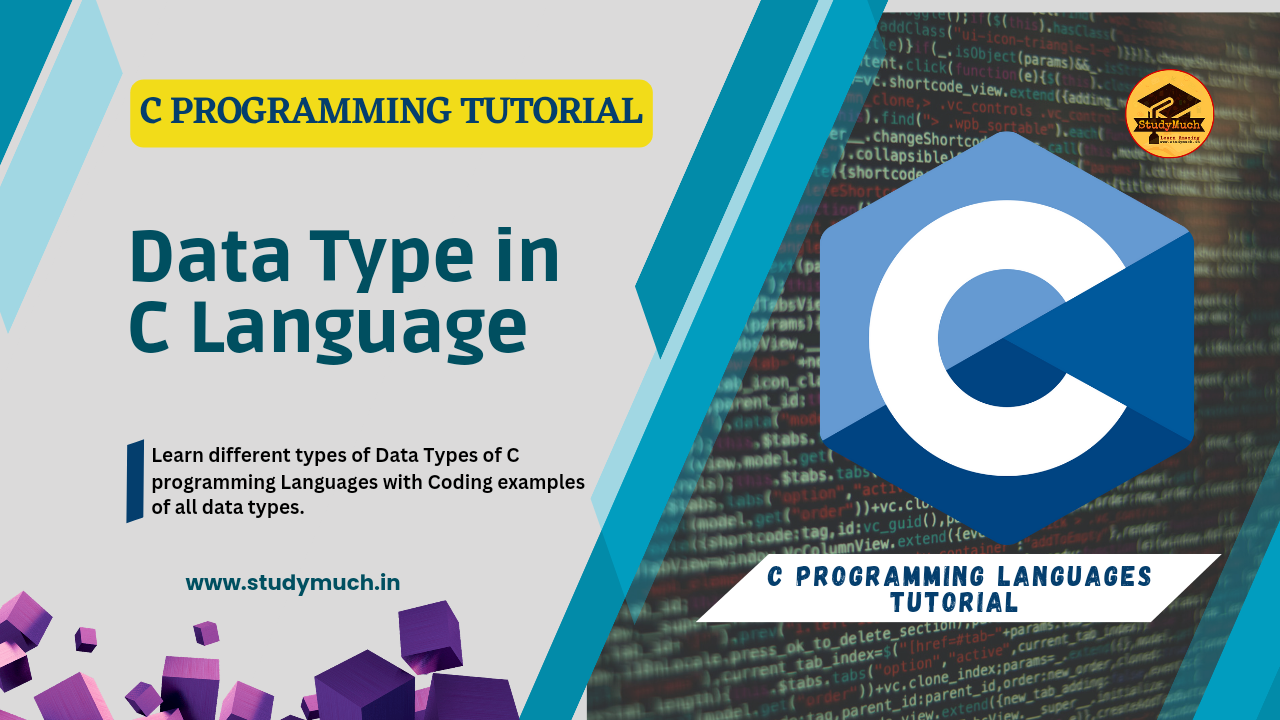
Data Type in C Language
Introduction:
Data types play a crucial role in programming as they define the type and size of data that can be stored in variables. In the C programming language, understanding data types is fundamental for efficient and error-free coding. In this blog post will provide an in-depth exploration of data types in C, along with examples to illustrate their usage.
What is Data Type in C Language?
In C, a data type is a classification or category that specifies the type of data that a variable can hold. It defines the range of values that the variable can take, the operations that can be performed on the variable, and the amount of memory space required to store the variable. The C programming language provides several built-in data types, each serving a specific purpose.
A data type specifies the type of data that a variable can store such as integer, floating, character, etc.
Here are the commonly used data types in C, with programming examples:
Integer Types:
-
int: Used to store whole numbers. Typically 4 bytes in size.
-
short int: Used to store smaller whole numbers. Typically 2 bytes in size.
-
long int: Used to store larger whole numbers. Typically 8 bytes in size.
-
unsigned int: Used to store positive whole numbers. Same size as int, but the range starts from 0.
Floating-Point Types:
-
float: Used to store single-precision floating-point numbers. Typically 4 bytes in size.
-
double: Used to store double-precision floating-point numbers. Typically 8 bytes in size.
Character Types:
-
char: Used to store a single character. Typically 1 byte in size.
Void Type:
-
void: Used to indicate the absence of a specific type. It is commonly used as a return type for functions that do not return a value.
Derived Types:
-
Arrays: Used to store a collection of elements of the same type.
-
Pointers: Used to store memory addresses.
-
Structures: Used to define a custom data type that can hold multiple variables of different types.
-
Unions: Similar to structures but can store only one value at a time.
Size of Data Type in Byte
Here’s a table summarizing the commonly used data types in C along with their respective sizes in bytes:
Data Types | Memory Size | Range |
char |
1 byte |
−128 to 127 |
signed char |
1 byte |
−128 to 127 |
unsigned char |
1 byte |
0 to 255 |
short |
2 byte |
−32,768 to 32,767 |
signed short |
2 byte |
−32,768 to 32,767 |
unsigned short |
2 byte |
0 to 65,535 |
int |
2 byte |
−32,768 to 32,767 |
signed int |
2 byte |
−32,768 to 32,767 |
unsigned int |
2 byte |
0 to 65,535 |
short int |
2 byte |
−32,768 to 32,767 |
signed short int |
2 byte |
−32,768 to 32,767 |
unsigned short int |
2 byte |
0 to 65,535 |
long int |
4 byte |
-2,147,483,648 to 2,147,483,647 |
signed long int |
4 byte |
-2,147,483,648 to 2,147,483,647 |
unsigned long int |
4 byte |
0 to 4,294,967,295 |
float |
4 byte |
|
double |
8 byte |
|
long double |
10 byte |
 |
Now, here you learn programming examples of Data Types in C language;
Integer Data Types:
C offers various integer data types with different ranges and memory sizes. These data types are commonly used for storing whole numbers.
a) int: The most commonly used integer data type in C is int. It typically uses 4 bytes of memory and can represent a range of values from -2,147,483,648 to 2,147,483,647. For example:
int age = 25;
b) short int: The short int data type uses 2 bytes of memory and represents a smaller range of values than int. It can hold values from -32,768 to 32,767. For example:
short int temperature = -10;
c) long int: The long int data type uses 8 bytes of memory and allows the storage of larger values than int. It can represent values from approximately -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807. For example:
long int population = 7894561230;
Floating-Point Data Types:
Floating-point data types are used to represent real numbers with fractional parts.
a) float: The float data type is used to store single-precision floating-point numbers. It typically uses 4 bytes of memory and has a precision of about 6 decimal places. For example:
float pi = 3.14159;
b) double: The double data type provides double-precision floating-point numbers. It uses 8 bytes of memory and has a higher precision than float, usually up to 15 decimal places. For example:
double gravity = 9.81;
Character Data Types:
In C, characters are represented using the char data type.
a) char: The char data type is used to store a single character. It uses 1 byte of memory and can hold any character from the ASCII character set. For example:
char name = 'A';
Other Data Types:
C provides additional data types to handle specific requirements.
a) unsigned: By adding the unsigned keyword before an integer type, you can create an unsigned variant of that type. Unsigned types can only represent non-negative values but have a larger positive range compared to their signed counterparts. For example:
unsigned int positiveNumber = 100;
b) void: The void data type is used to indicate the absence of a specific type. It is often used as a return type for functions that do not return any value. For example:
void printHello() { Â printf("Hello, world!"); }
It’s important to remember that C is a statically-typed language, which implies that before being used, variables must be defined with the appropriate data types. This enables type verification and memory allocation during compilation by the compiler.
For creating effective and error-free code in C, it is essential to understand data types. The proper use of memory is ensured, and unexpected behaviour or data loss during operations is prevented by selecting the right data type for a variable.
Conclusion:
Understanding data types in C is essential for effective programming. By selecting appropriate data types, you can optimize memory usage and ensure the correctness of your programs. This blog post has covered the most commonly used data types in C, including integers, floating-point numbers, characters, and additional types. By exploring and experimenting with these data types, you’ll gain a solid foundation for C programming and be better equipped to handle various coding challenges.
Learn Related;
0 Comments