Draw a Rainbow with Python Code
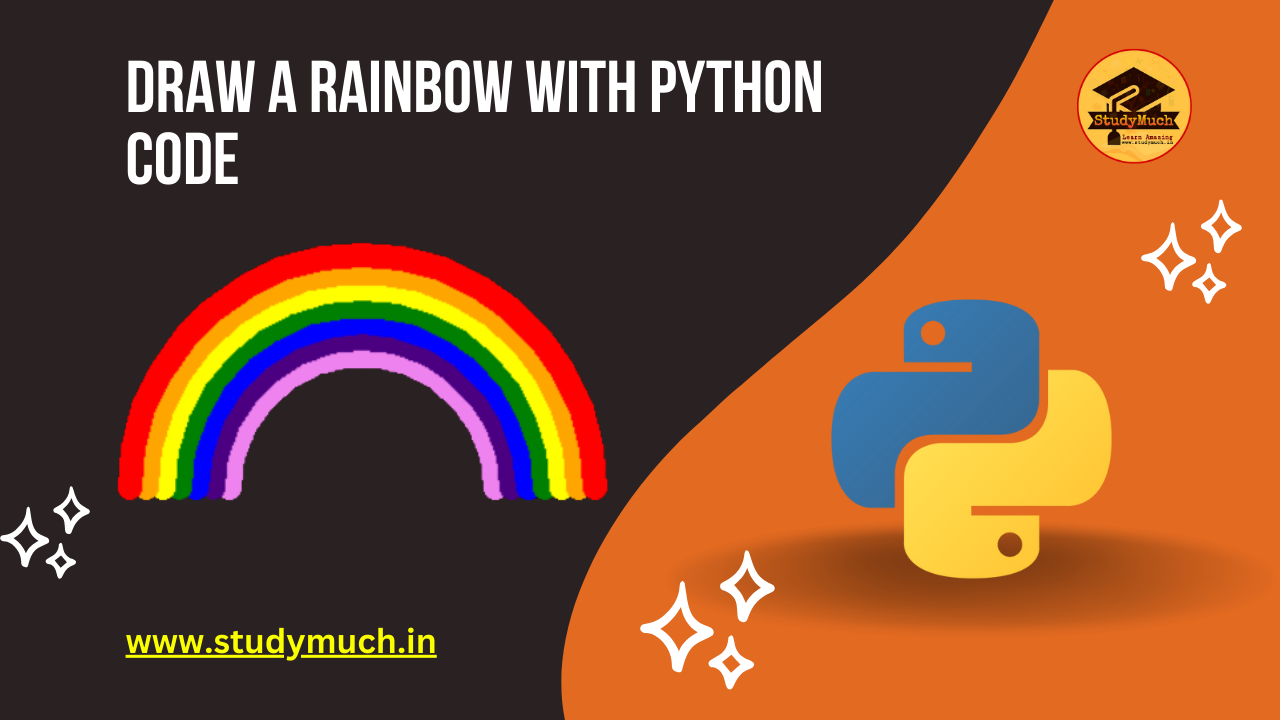
Draw a Rainbow with Python Code
Rainbows are a natural wonder, a beautiful display of colors that often appear after a rain shower. But have you ever wanted to create your own rainbow? Well, you’re in luck! In this blog post, we will show you how to draw a Rainbow with Python Code. We’ll break down the code step by step and explain the purpose of each function.
Prerequisites
Before we dive into the code, make sure you have Python installed on your computer. You’ll also need the Turtle graphics library, which is included in the standard library of Python, so there’s no need to install it separately.
The Python Code
import turtle # Create a screen and a turtle sc = turtle.Screen() pen = turtle.Turtle() # Define a function to draw a semi-circle def semi_circle(col, rad, val): pen.color(col) pen.circle(rad, -180) pen.up() pen.setpos(val, 0) pen.down() pen.right(180) # Define the colors of the rainbow col = ['violet', 'indigo', 'blue', 'green', 'yellow', 'orange', 'red'] # Set up the screen sc.setup(700, 700) sc.bgcolor('black') pen.right(90) pen.width(15) pen.speed(10) # Draw the rainbow for i in range(7): semi_circle(col[i], 10* (i + 8), -10* (i + 1)) # Hide the turtle pen.hideturtle()
Understanding the Code
Now, let’s break down the code step by step and understand how it works.
- We import the turtle module to create our drawing canvas.
- We create a screen (sc) and a turtle (pen) object. The turtle will be used to draw the rainbow on the screen.
- The semi_circle function takes three parameters: col (color of the semi-circle), rad (radius of the semi-circle), and val (position to move the turtle to after drawing). This function is responsible for drawing each colored semi-circle of the rainbow.
- We define a list of colors (col) representing the colors of the rainbow in the correct order.
- We set up the screen with a size of 700×700 pixels and a black background.
- We position the turtle to face upward (pen.right(90)) and set its width and drawing speed.
- In the loop, we iterate through the colors and draw semi-circles with increasing radii and adjusted positions to create the rainbow effect.
- Finally, we hide the turtle (pen.hideturtle()) to display only the rainbow.
Turtle Graphics
In the provided Python code for drawing a rainbow, the turtle functions are used to create and control the turtle graphics. Here’s a brief explanation of how the turtle functions are utilized in the code:
- turtle.Screen(): This function creates a screen or canvas for drawing. It is assigned to the variable sc, allowing you to set properties of the drawing area, such as size and background color.
- turtle.Turtle(): This function creates a turtle object for drawing on the canvas. It is assigned to the variable pen, which you can use to control the turtle’s movements and drawing actions.
- pen.color(col): This method sets the color of the turtle’s drawing pen to the specified color (col).
- pen.circle(rad, -180): This method makes the turtle draw a semi-circle with the given radius (rad) and an angle of -180 degrees, which creates the curved shape of the rainbow.
- pen.up(): This method lifts the turtle’s drawing pen, allowing the turtle to move without drawing.
- pen.setpos(val, 0): This method moves the turtle to the specified position (val, 0) without drawing a line.
- pen.down(): This method lowers the turtle’s drawing pen, allowing it to draw when it moves.
- pen.right(180): This method turns the turtle to the right by 180 degrees, ensuring that the semi-circles are oriented correctly.
- pen.width(15): This method sets the width of the turtle’s drawing pen to 15 units, making the lines thicker.
- pen.speed(10): This method sets the drawing speed of the turtle to 10, controlling how fast the turtle moves and draws.
- pen.hideturtle(): This method hides the turtle cursor after drawing the rainbow, so only the rainbow itself is visible.
These turtle functions are essential for creating and controlling the turtle graphics used to draw the colorful semi-circles that make up the rainbow in the code. They allow you to specify colors, draw shapes, move the turtle, and control its appearance and behavior on the canvas.
Running the Code
To run this code, you can create a Python file (e.g., rainbow.py) and paste the code into it. Then, simply execute the Python script, and a window will pop up displaying your beautiful rainbow!
In conclusion, creating a rainbow with Python code is not only a fun project but also a great way to learn about programming and graphics. You can customize the colors, sizes, and positions to create your unique rainbow masterpiece. Have fun coding, and may your day be as colorful as your rainbow!
Learn More;
0 Comments