Operators in Java
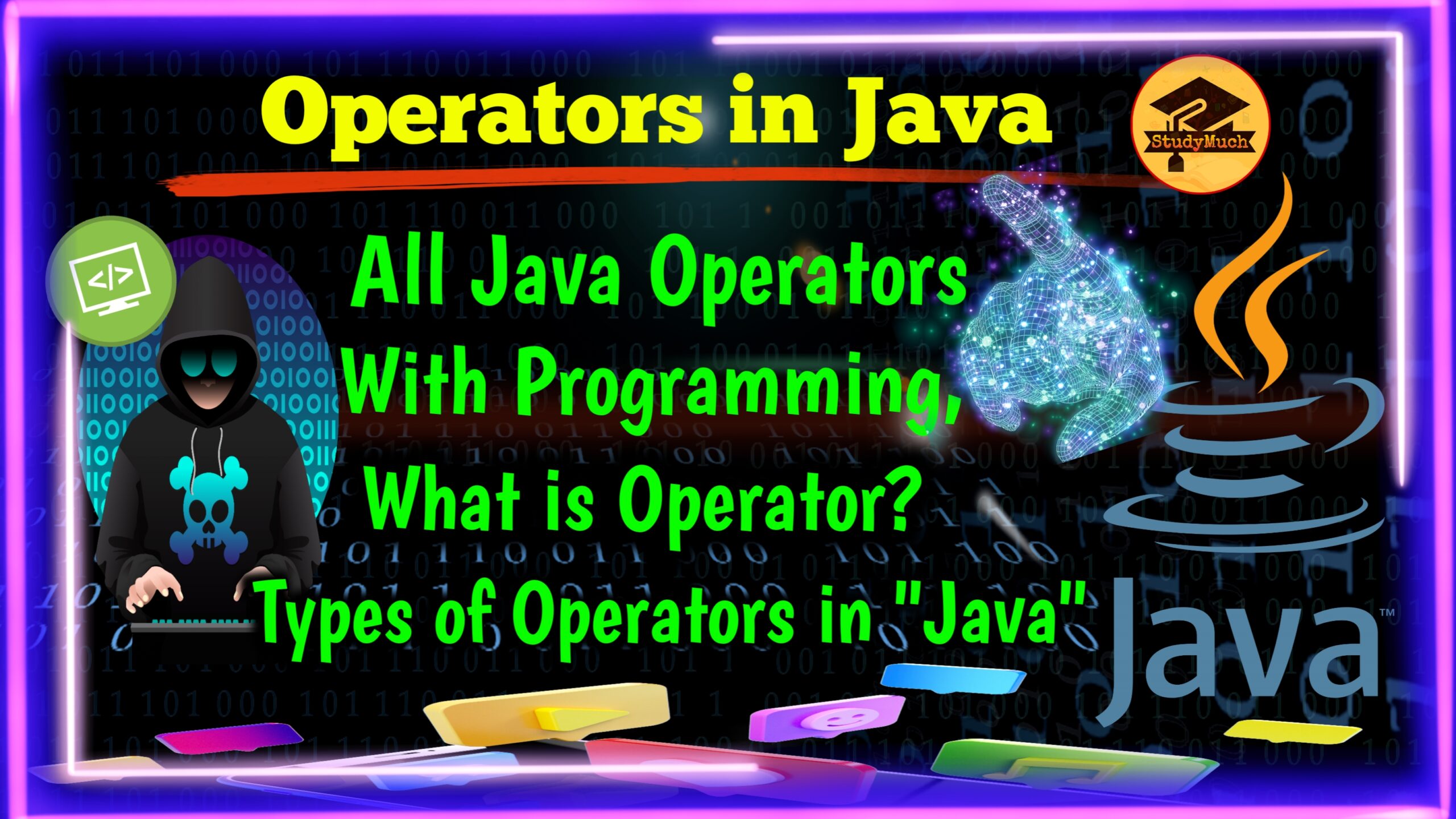
Operators in Java
In this tutorial you will learn and discuss what is Java Operator and all types of Operators available in java and also see their uses in expression, here you read all the Operators with programming examples.
What is Java Operator?
Operator in Java is a symbol that is used to perform operations. For example: +, -, /, * etc.
An operator is a symbol that tells the computer to perform certain mathematical or logical manipulations, Operators are used in programs to manipulate data variable. They usually form a part of mathematical or logical expression.
Types of Operators in Java;
There are many types of Operators in Java are given below;
- Arithmetic Operator
- Assignment Operator
- Logical Operator
- Relational Operator
- Conditional Operator
- Bitwise Operator
- Increment & Decrement Operator
- Special Operator
Define below all the operators of Java, with expression and programming examples also.
Arithmetic Operator
The operator that are used to perform arithmetic operation, such as addition, subtraction, multiplication, division etc. are called the Arithmetic Operators.
Arithmetic Operator are used to construct mathematical expression as in algebra. All the arithmetic operators in Java are given below in table.
Arithmetic Operator |
|
Operator |
Name and meaning |
+ |
Addition or Unary Plus |
– |
Subtraction or Unary Minus |
* |
Multiplication |
/ |
Division |
% |
Modulo Division |
Programming Example Arithmetic Operator;
package com.company; public class javaoperators{ public static void main (String[] args) { //Arithmetic operator int a = 7, b = 3; int sum = a + b; System.out.print ("sum of a and b is; "); System.out.println(sum); int c = 8; int d = 20 - c; System.out.print ("Subtraction of d with c is;"); System.out.println(d); } } |
Output is;
Assignment Operator
Assignment operator is one of the most common operator. Assignment operator are used to assign the value of an expression to a value. We have seen the usual assignment operator is “=”.
We also used Shorthand Assignment Operators is Java. The shorthand operator example; += means ‘add y+1 to x’ or ‘increment x by y+1’. For y = 2 the statement become x += 3;
Some of the commonly used shorthand assignment operators are given below in table.
Shorthand Assignment Operator |
||
Shorthand Operator |
Shorthand Statement |
Meaning |
+= |
a + = 2 |
a = a + 2 |
-= |
a – = 2 |
a = a – 2 |
*= |
a * = 2 |
a = a * 2 |
/= |
a / = 2 |
a = a / 2 |
%= |
a % = 2 |
a = a % 2 |
Programming Example Assignment Operator;
package com.company; public class javaoperators{ public static void main (String[] args) { //Assignment operator int a = 4; a += 4; System.out.print ("The sum of a and a is; "); System.out.println(a); int b = 5; b *= 5; System.out.print ("Multiplication of b and b is; "); System.out.println(b); } } |
Output is;
Logical Operator
A logical operator is a symbol are used to connect two or more expression such that the value of the compound expression produced depends only on that of the original expressions.
The logical operators && and || are used when we want to form compound conditions by combining two or more relations.
Java has three Logical Operators which are given in below table.
Logical Operator |
|
Operator |
Name and meaning |
&& |
Logic AND |
|| |
Logic OR |
! |
Logical NOT |
Programming Example of Logical Operator;
package com.company; public class javaoperators{ public static void main (String[] args) { //Logical operator int a = 4; int b = 10; System.out.println(a>b && b<a); System.out.println(b>a || a<b); System.out.println ( 110>10 && 10<110); } } |
Output is;
Relational Operator
The Relational operator is also known as “Compare operator”, are used to compare two operators. They are used to find the relationship between two operant and hance are called Relational operator.
Java support six relational operators, and these operators and their meanings are given below in table.
Relational Operators | |
Operator |
Meaning |
< | Less than |
< = | Less than or equal to |
> | Greater than |
> = | Greater than or equal to |
= = | Equal to |
! = | Not equal to |
Programming Example of Relational Operator;
package com.company; public class javaoperators{ public static void main (String[] args) { //Relational operator int a = 4; int b = 10; System.out.println(a==b); System.out.println(a<b); System.out.println(a>=b); System.out.println(a!=b); System.out.println(10 == 10); } } |
Output is;
Conditional Operator
The conditional operator is also known as “Ternary operator” as the name indicate an operator that operate three operands in called ternary operator.
Ternary operator is used as one liner replacement for if, else statement and use d a slot in java programming. It is the only conditional operator which takes three operands.
The general form of conditional operator is; exp 1 ? exp2 : exp3
Where exp1, exp2 and exp3 are expressions.
The operator ? : works as follows: exp 1 is evaluated first. If it is non-zero (true), then expression exp2 is evaluated as becomes the value of the conditional expression. It exp1 is false, exp3 is evaluated and its value become the value of the conditional expression.
Note; that only one of the expressions (either exp2 or exp3) is evaluated.
Programming Example of Conditional Operator;
package com.company; public class javaoperators{ public static void main (String[] args) { //Conditional operator int a = 4; int b = 3; int c = 6; System.out.println (a<c && c>b || b<a); System.out.println (b>a || c<a && b<c); } } |
Output is;
Bitwise Operator
The bitwise operator is the operator used to perform the operations on the data at the bit-level.
Java has a distinction of supporting special operators known as bitwise operators for manipulation of data at values of bit level. These operators are used for testing the bits, or shifting them to the right or left.
NOTE; Bitwise Operator may not be applied to float or double.
The list of bitwise operators in below table;
Bitwise Operators | |
Operator | Meaning and Name |
& | Bitwise AND |
! | Bitwise OR |
^ | Bitwise exclusive OR |
~ | One’s complement |
<< | Shift left |
>> | Shift right |
>>> | Shift right with zero fill |
Programming Example of Bitwise Operator;
package com.company; public class javaoperators{ public static void main (String[] args) { //Bitwise operator int a = 4; int b = 7; System.out.println(a&b); System.out.println(a|b); System.out.println(a<<b); System.out.println(a^b); } } |
Output is;
Increment and Decrement Operator
Increment and decrement operator are unary operators that add or subtract one, to or form their operand, respectively. They are commonly implemented in Java.
The Increment operator is written as ++ and the Decrement operator is written as – –. The increment operator increases and the decrement operator decreases, the value of its operand by 1.
Programming Example of Increment & Decrement Operator;
package com.company; public class javaoperators{ public static void main (String[] args) { //Increment & Decrement operator int a = 4; int b = 7; System.out.print ("Increment Op; "); System.out.println(++a); System.out.print ("Decrement Op; "); System.out.println(--b); } } |
Output is;
Special Operator
Java supports some special operators of interest such as “instanceof” and Dot operator (.).
Instanceof Operator
The instanceof is an object reference operator and return true if the object on the left-hand side is an instance of the class given on the right-hand side. This operator allows us to determine whether the object belongs to a particular class or not.
Example: Person Instanseof student
Is true if the object person belongs to the class student; otherwise, it is false.
Dot Operator (.)
The Dot operator is used to access the instance variable and method of class object.
Example:
personal. age // Reference to the variable age
personal. salary ( ) // Reference to the method salary ( )
So, in this tutorial you learned, all about the Java Operator, what is operator, types of operators with programming examples of all the operators. I hope you read this tutorial well and learn something new from this Java Tutorial. If you have any doubt from this tutorial then you can ask in the comment section.
Read Also –
5 Comments
Manish · August 6, 2022 at 7:12 am
Awesome Brother ☺️
StudyMuch · August 6, 2022 at 12:59 pm
Thanks bro… 👍
zoritoler imol · October 29, 2022 at 8:07 pm
I have not checked in here for a while since I thought it was getting boring, but the last several posts are great quality so I guess I?¦ll add you back to my daily bloglist. You deserve it my friend 🙂
Vytvořit osobní účet · April 14, 2023 at 12:20 am
Your article helped me a lot, is there any more related content? Thanks!
expedited · May 8, 2023 at 7:05 pm
Howdy wouⅼd you mind ѕtating which blog platform you’re
using? I’m going to start my own blog soon but I’m having a harɗ
timе making a decision between BloɡEngine/Wordprеss/B2evolution and Drupal.
The reason I ask is Ƅecause your design seems different
then most blogs and I’m looking for something completely unique.
P.Ѕ Sorry for being off-topic but I had to ask!