Python Identify Operators
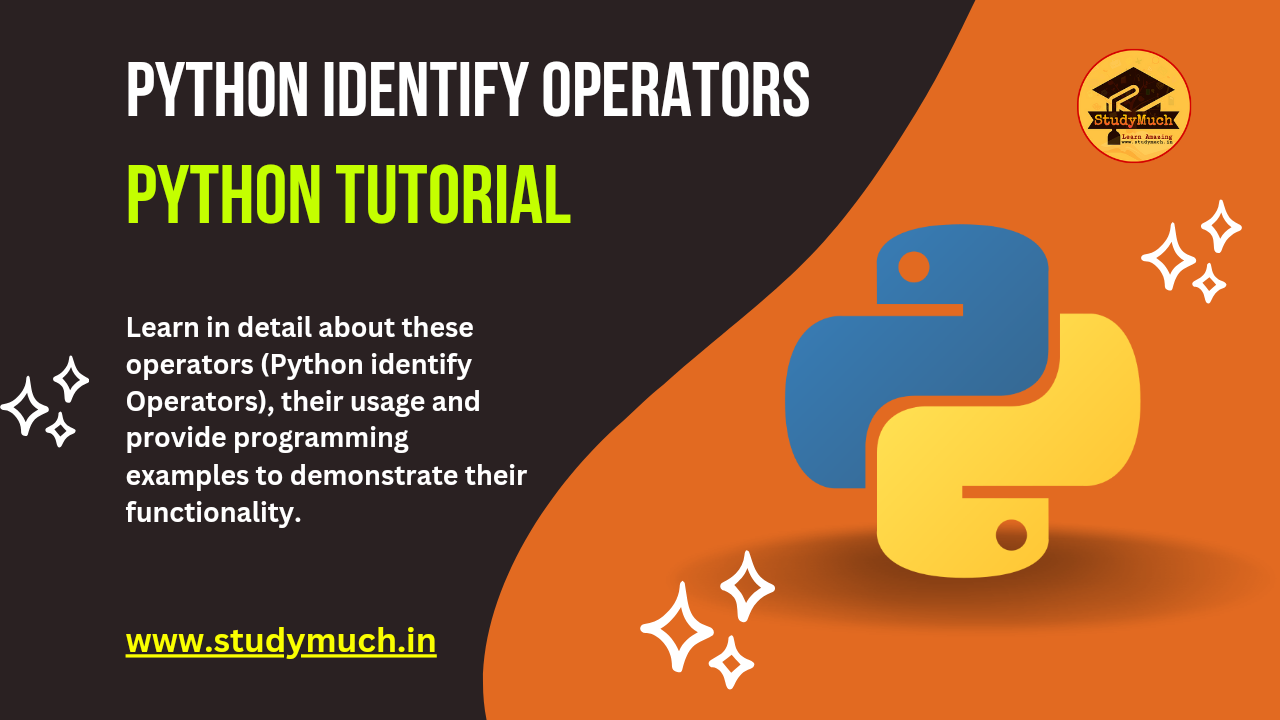
Python Identify Operators; “is and is not”
When working with Python, you’ll often encounter scenarios where you need to compare objects for identity rather than equality. In such cases, Python provides two identity operators: is and is not. These Python Identify Operators allow you to check whether two variables or objects reference the same memory location, indicating the same underlying object. In this blog post, we will learn in detail about these operators (Python identify Operators), their usage and provide programming examples to demonstrate their functionality.
The “is” Operator
The is operator
is used to test if two variables or objects refer to the same memory location. It checks for object identity rather than object equality. The “is operator” returns True if both operators point to the same objects.
Here’s the basic syntax:
x is y
Here, x and y are the variables or objects you want to compare.
Example 1: Comparing Variables
a = [1, 2, 3] b = a # Both a and b reference the same list object result = a is b print(result) # True, because a and b point to the same object
Example 2: Comparing Objects
class MyClass: def __init__(self, value): self.value = value obj1 = MyClass(42) obj2 = obj1 # Both obj1 and obj2 reference the same instance result = obj1 is obj2 print(result) # True, because obj1 and obj2 refer to the same instance
The “is not” Operator
The is not operator, on the other hand, checks if two variables or objects do not refer to the same memory location. It is the negation of the is operator. The “is not” operator returns True if both operators do NOT point to the same object.
Here’s the basic syntax:
x is not y
Example 1: Comparing Variables
x = [1, 2, 3] y = [1, 2, 3] # Two different list objects with the same values result = x is not y print(result) # True, because x and y point to different objects
Example 2: Comparing Objects
class MyClass: def __init__(self, value): self.value = value obj1 = MyClass(42) obj2 = MyClass(42) # Two different instances with the same attribute value result = obj1 is not obj2 print(result) # True, because obj1 and obj2 are distinct instances
When to Use “is and is not”
- Immutable Objects: For immutable objects like integers, strings, and tuples, “is“
and “is not” are generally not required. You can use == and != to compare their values.
x = 5 y = 5 result = x is y # May or may not be True, as Python caches small integers print(result)
- Mutable Objects: Use is and is not when comparing mutable objects like lists, dictionaries, and custom classes. These operators ensure that you are checking for the same object in memory rather than just equivalent values.
-
Singletons: In some cases, you might want to check if a variable “is” referring to a specific singleton object, like None or a custom singleton instance. “is” and “is not” are suitable for this purpose.
Conclusion;
In Python, the is
and is not
operators are essential tools for comparing object identities. They help you determine whether two variables or objects refer to the same memory location. When working with mutable objects or checking specific singleton instances, these operators are important for accurate comparisons. However, for immutable objects, it is generally more appropriate to use the equality operators == and != to compare their values. Understanding the difference between identity and equality is fundamental to writing strong Python code. So, in this tutorial you have learn about the Python Identify Operators, I hope you understood this welled, but if you have any doubt you can ask with any hesitation.
Learn More;
0 Comments