Python List
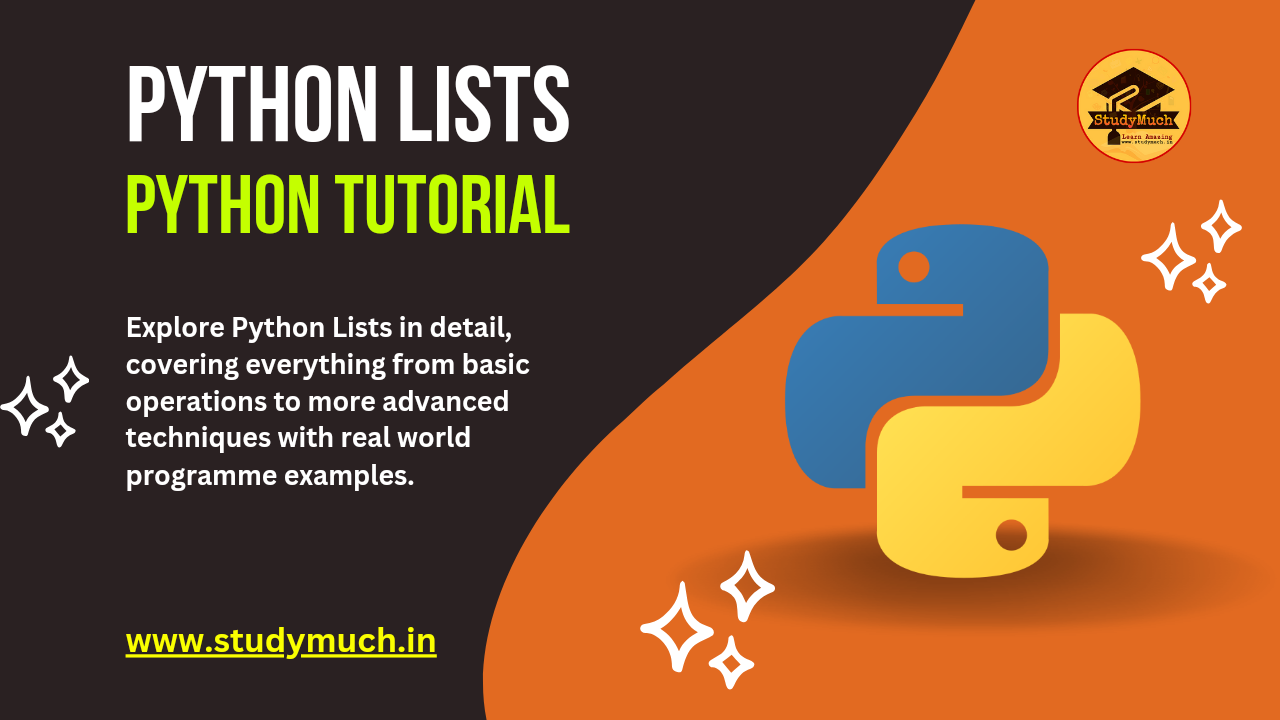
Python List: A Comprehensive Guide
Lists are an essential data structure in Python, and they play a fundamental role in many programming tasks. A list is a versatile container that can hold a collection of items, allowing you to perform various operations on them. In this comprehensive guide, we’ll explore Python Lists in detail, covering everything from basic operations to more advanced techniques.
What is a Python List?
A Python list is an ordered collection of elements, where each element can be of any data type. Lists are defined using square brackets ([]) and can contain zero or more elements. Here’s a basic example of a Python list:
Look! Here a basic example of Python List;
fruits = ['apple', 'banana', 'cherry'] print(fruits)
Now, let’s look at different aspects of Python lists:
Indexing
Indexing is used to access the items of a list. You can access individual elements in a list using their index. Python uses zero-based indexing, meaning the first element has an index of 0, the second has an index of 1, and so on. To access an element, use square brackets with the desired index:
fruits = ['apple', 'banana', 'cherry'] print(fruits[0]) # Access the first element (1) print(fruits[2]) # Access the first element (2)
Negative Indexing
Python also supports negative indexing; it is used to access the items of a list using negative number. which allows you to access elements from the end of the list. Where -1 refers to the last items, -2 refers to the second to the last item, and so on.
fruits = ['apple', 'banana', 'cherry'] print(fruits[-1]) # Access the last element (3) print(fruits[-2]) # Access the second-to-last element (2)
Range of Indexes
You can access a range of elements in a list by specifying a start and end index within square brackets separated by a Colon (:). Simply separate tow indexes using the colon. the first index is the start of the range, while the second index is the end of the range (not included).
fruits = ['Apple', 'Banana', 'Cherry', 'Mango'] f = fruits[1:3] print(f) #Output - ['Banana', 'Cherry']
If you don’t specify the first index, the range starts from index 0.
fruits = ['Apple', 'Banana', 'Cherry', 'Mango'] f = fruits[:2] print(f) #Output - ['Apple', 'Banana']
If you don’t specify the last index, the range ends with the last item of the list. In this case, the range includes the last item.
fruits = ['Apple', 'Banana', 'Cherry', 'Mango'] f = fruits[2:] print(f) #Output - ['Cherry', 'Mango']
Adding Items to a List
To add an item to the end of a list, you can use the append() method: In this example, we will add “Mango” to our fruits list.
fruits = ['Apple', 'Banana', 'Cherry'] fruits.append("Mango") print(fruits) # ['Apple', 'Banana', 'Cherry', 'Mango']
You can also use the insert() method to add an item at a specific index:
fruits = ['Apple', 'Banana', 'Cherry'] # Insert "Mango" at index 1 fruits.insert(1, "Mango") # Insert "Litchi" at index 3 fruits.insert(3, "Litchi") print(fruits) #['Apple', 'Mango', 'Banana', 'Litchi', 'Cherry']
Deleting Items from a List
To remove an specified item from a list by value, use the remove() method:
fruits = ['Apple', 'Banana', 'Cherry', 'Mango'] fruits.remove("Banana") print(fruits) #['Apple', 'Cherry', 'Mango']
To removes the last item from a list, you can use pop() method.
fruits = ['Apple', 'Banana', 'Cherry', 'Mango'] fruits.pop() print(fruits) #['Apple', 'Banana', 'Cherry']
To delete a specified index, you can use del keyword.
fruits = ['Apple', 'Banana', 'Cherry', 'Mango'] del fruits[1] print(fruits) #['Apple', 'Cherry', 'Mango']
Getting the Length of a List
You can find the number of elements in a list using the len() function. look the programming example given below;
fruits = ['Apple', 'Banana', 'Cherry', 'Mango'] print(len(fruits)) #4
Changing an Item’s Value
To change an item’s value, access the index first and use the assignment operator. You can modify the value of an element in a list by assigning a new value to its index:
fruits = ['Apple', 'Banana', 'Cherry'] fruits[2] = "Mango" print(fruits) #['Apple', 'Banana', 'Mango']
Checking if an Item Exists
To check if an item is present in a list, you can use the in operator:
fruits = ['Apple', 'Banana', 'Cherry'] print("Banana" in fruits) #True print("Mango" in fruits) #False
Extending a List
You can extend one list with the elements of another using the extend() method or the + operator:
list1 = [1, 2, 3] list2 = [4, 5, 6] list1.extend(list2) # Appends elements of list2 to list1 # Alternatively: list1 += list2 print(list1) [1, 2, 3, 4, 5, 6]
Looping Through a List
You can iterate through the elements of a list using a for loop, looping through a list basically means accessing all its items one-by-one. The for loop is used to loop through a list;
fruits = ['Apple', 'Banana', 'Cherry'] for fruit it fruits: print(fruit) # Prints each element on a separate line
Conclusion;
Python lists are versatile and essential data structures for various programming tasks. They allow you to store, access, modify, and manipulate collections of data efficiently. Understanding the operations and methods available for working with lists is crucial for any Python programmer. Now that you have a comprehensive understanding of Python lists, I hope you have understood this tutorial, but you have any doubts, then ask in the comment section.
Learn More;
0 Comments