Python Number Methods
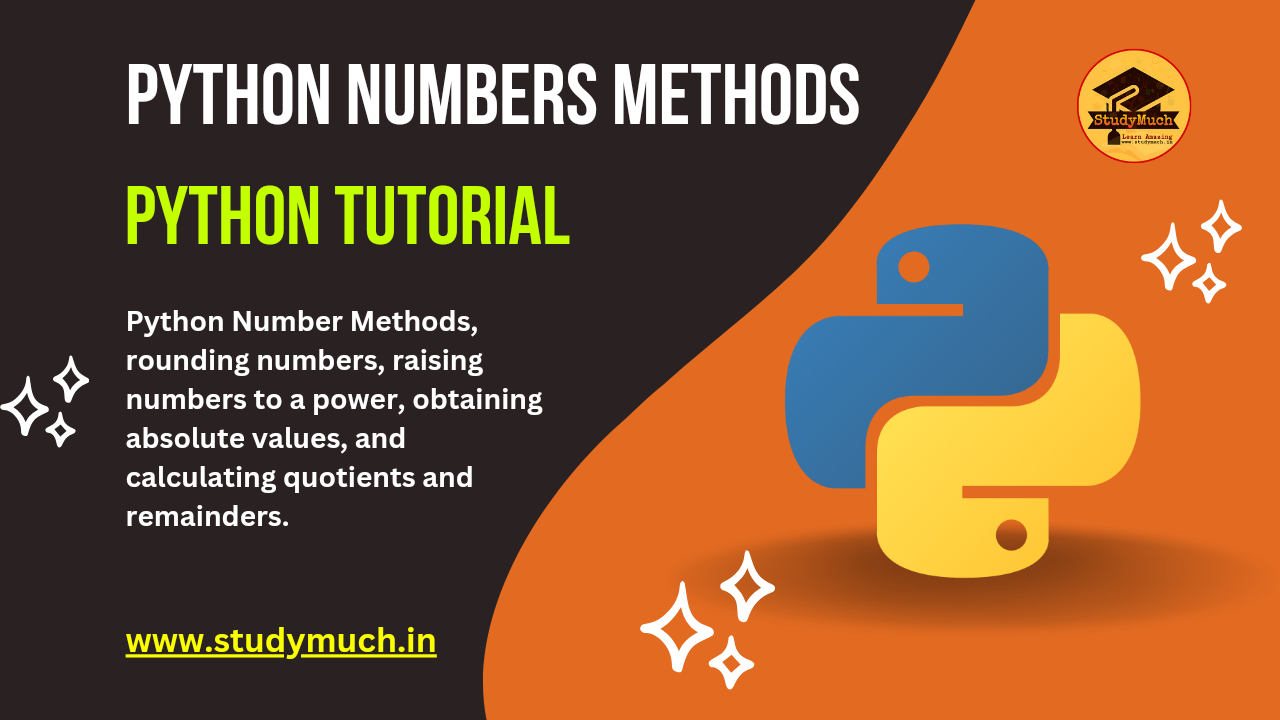
Python Number Methods: A Comprehensive Guide
Python, a versatile and widely-used programming language, comes with a plethora of built-in methods that facilitate various mathematical operations. In this blog post, we’ll dive into the world of Python number methods, exploring how they can be utilized for tasks such as rounding numbers, raising numbers to a power, obtaining absolute values, and calculating quotients and remainders.
Rounding a Number
Rounding numbers is a common operation in many applications, whether it’s for simplifying results or displaying values in a user-friendly format. Python provides several methods to achieve different types of rounding.
Round()
The round() function is used to round a floating-point number to the nearest integer or to a specified number of decimal places. It follows the conventional rounding rules where values ending in .5 are rounded to the nearest even number.
num = 3.75 rounded_num = round(num) print(rounded_num) # Output: 4 num = 3.14159 rounded_num = round(num, 2) print(rounded_num) # Output: 3.14
Rising a Number to a Power
Raising a number to a power, also known as exponentiation, is a fundamental mathematical operation that involves multiplying a number (the base) by itself a certain number of times (the exponent). The exponent represents how many times the base should be multiplied by itself. The result of this operation is a new number, often referred to as the “power” or “exponential value.”
For example, in Python, you can use the ** operator or the pow() function to raise a number to a power:
base = 2 exponent = 3 result = base ** exponent print(result) # Output: 8
You can also use the built-in pow() function to achieve the same result:
result = pow(base, exponent) print(result) # Output: 8
Getting Absolute Value
The absolute value of a number is its distance from zero on the number line. Python’s abs() function returns the absolute value of a given number.
For example, he absolute value of 21 is 2 and the absolute value of -8 is 8.
num = -5 absolute_value = abs(num) print(absolute_value) # Output: 5
Getting Quotient and Remainder
To obtain both the quotient and remainder of a division operation, Python provides the divmod() function. This can be particularly useful when you need to perform division and also capture the remainder for further calculations.
In this example, 17 is divided by 5 therefore the quotient is 3 and the remainder is 2.
dividend = 17 divisor = 5 quotient, remainder = divmod(dividend, divisor) print(f"Quotient: {quotient}, Remainder: {remainder}") # Output: Quotient: 3, Remainder: 2
Conclusion
Python number methods provide a wide range of functionalities to handle various mathematical operations. Whether you’re working with integers, exponents, absolute values, or division operations, Python’s built-in functions and operators provide an efficient and intuitive way to perform these calculations. Using these capabilities, you can streamline your code and focus on solving more complex problems in your applications. So go ahead and explore the power of Python’s number methods in your coding endeavours!
Learn More;
- Learn Tutorial of Java programming.
- Learn Tutorial of HTML and CSS.
- Learn Tutorial of C language.
- Learn about Computer Fundamental.
0 Comments