Python Numbers
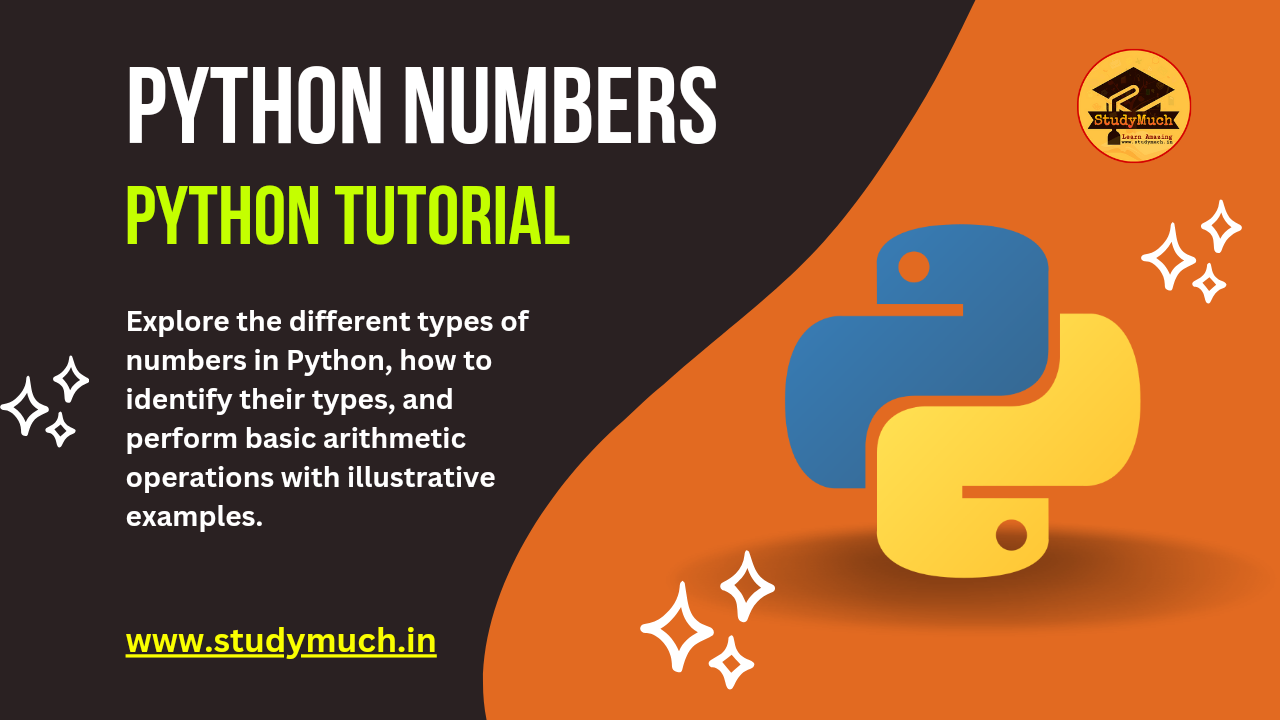
Python Numbers: A Comprehensive Guide to Numeric Data Types and Operations
Numbers are an essential foundation of programming, allowing us to perform calculations, manipulate data, and solve complex problems. In Python, a versatile and widely used programming language, numbers are represented by a variety of data types, each serving specific purposes. In this guide, we’ll explore the different types of numbers in Python, how to identify their types, and perform basic arithmetic operations with illustrative examples.
Types of Numeric Data in Python
Python supports several types of numeric data, each designed to handle different kinds of numbers. The main types are:
- Integers (int): Integers are whole numbers, positive or negative, without any decimal points
- Floating-Point Numbers (float): Floating-point numbers are numbers with decimal points or numbers expressed in scientific notation.
- Complex Numbers (complex): Complex numbers consist of a real part and an imaginary part, typically written as a + bj, where a and b are real numbers, and j is the imaginary unit.
Let’s take a closer look at each type with examples:
Example of Integer Data Type;
Integers (int)
# Examples of integers a = 10 b = -5 c = 0
Example of Floating-Point Numbers (float)
# Examples of floating-point numbers x = 10.02 y = -5.53 z = 5e2 # 5 * 10^2 = 500.0
Example of Complex Numbers (complex)
# Examples of complex numbers p = 2 + 3j q = -1j r = 1.5 - 2j
Identifying numeric data types
Python provides built-in functions for determining the type of a given numeric value. The type()
function can be used to identify the type of a number:
num = 42 num_type = type(num) print(num_type) # Output: <class 'int'>
Basic Arithmetic Operations
Python allows us to perform fundamental arithmetic operations on numeric data types. These operations include addition, subtraction, multiplication, and division.
Addition;
# Addition of numbers a = 10 b = 20 sum_ab = a + b print(sum_ab) # Output: 30
Subtraction;
# Subtraction of numbers x = 5.5 y = 3.0 diff_xy = x - y print(diff_xy) # Output: 2.5
Multiplication;
# Multiplication of numbers p = 2j q = 4j product_pq = p * q print(product_pq) # Output: (8j)
Division;
# Division of numbers m = 15 n = 3 div_mn = m / n print(div_mn) # Output: 5.0
Putting It All Together
Now, let’s combine the knowledge of numeric data types and arithmetic operations to solve a real-world problem. Suppose we want to calculate the total cost of purchasing a certain quantity of items at a given price per item:
quantity = 25 price_per_item = 2.5 total_cost = quantity * price_per_item print("Total cost:", total_cost) # Output: Total cost: 62.5
Getting the Type of Numerical Value;
In Python, it’s essential to know the type of data you’re working with, especially when dealing with numerical values. The type()
function is a powerful tool that allows you to determine the specific data type of a given value. We can get the specific data types of number using the type() function.
#specifying data types of number. a = 3 b = 23 c = 41.2 d = 5.21 e = 7.21j f = 3.33j print(type(a)) #output: <class 'int'> print(type(b)) #output: <class 'int'> print(type(c)) #output: <class 'float'> print(type(d)) #output: <class 'float'> print(type(e)) #output: <class 'complex'> print(type(f)) #output: <class 'complex'>
Putting it All Together
Now, let’s create a simple function that takes a numerical value as an argument and prints out its data type:
def print_data_type(value): data_type = type(value) print(f"Data Type of {value} is: {data_type}") # Test the function with various numerical values print_data_type(42) # Output: Data Type of 42 is: <class 'int'> print_data_type(3.14) # Output: Data Type of 3.14 is: <class 'float'> print_data_type(2 + 3j) # Output: Data Type of (2+3j) is: <class 'complex'>
Conclusion;
So, here you have learned Python Numbers and also learned that understanding numeric data types and performing basic arithmetic operations is important for any programmer. Python’s versatile support for integers, floating-point numbers, and complex numbers provides the flexibility to work with a wide range of mathematical scenarios. Armed with this knowledge, you are now able to effectively manipulate numerical data and solve various computational challenges in your coding journey.
Also Learn;
- Learn Data Types in Python.
- Learn variable in Python.
- Learn statement in Python.
- Learn Tutorial of Java.
- Learn Tutorial of JavaScript.
0 Comments