Python Tuple
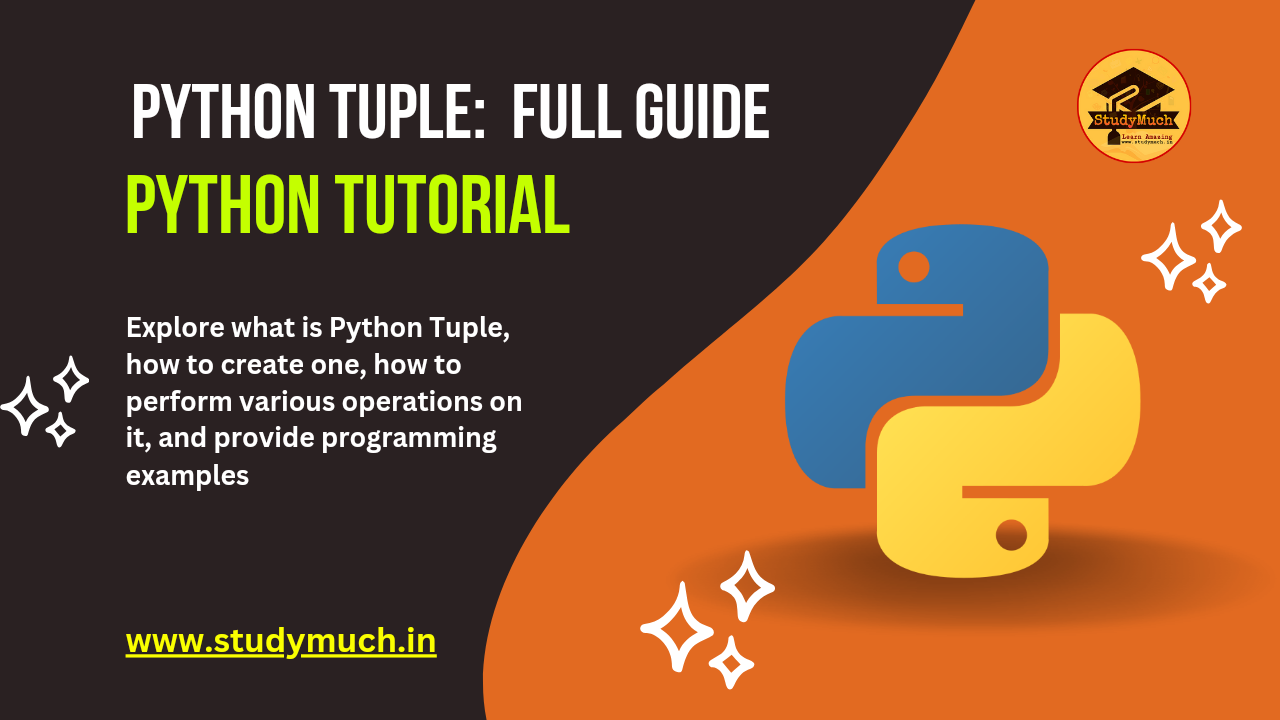
Python Tuple: A Comprehensive Guide
Tuples are a fundamental data structure in Python, and they play an important role in many aspects of Python programming. In this comprehensive guide, we will explore what is Python Tuple, how to create one, how to perform various operations on it, and provide programming examples to illustrate their uses.
What is a Python Tuple?
A tuple is an ordered and immutable collection of elements enclosed within parentheses (). Unlike lists, which use square brackets [], tuples cannot be modified once created. This immutability makes tuples suitable for situations where you need a collection of values that should not change during the program’s execution.
Creating a Tuple
Creating a tuple in Python is simple. You can define a tuple by placing a comma-separated sequence of elements within parentheses (). Here’s how you can create a tuple.
Example;
tuples = ('Rabbit', 9, 'Tiger', True) print(tuples)
You can also create a tuple without parentheses. Python will interpret the comma-separated values as a tuple:
x_tuple = 4, 5, 'world', 9 print(x_tuple)
Indexing in Tuples
Tuples are ordered collections, which means you can access their elements using indices. Python uses a zero-based index system, where the first element has an index of 0, the second has an index of 1, and so on. You can access tuple elements using square brackets and the index.
Look Example;
tuples = (1, 'Hello', 3, 'Python', 3.14) print(tuples[0]) # Access the first element (1) print(tuples[3]) # Access the third element (4)
Negative Indexing
Python also supports negative indexing, which allows you to access elements from the end of the tuple. The last element has an index of -1, the second-to-last has an index of -2, and so on.
Look Example;
tuples = (1, 'Hello', 3, 'Python', 3.14) print(tuples[-1]) # Access the last element (3.14) print(tuples[-4]) # Access the second element (Hello)
Range of Indexes (Slicing)
You can access a range of elements in a tuple by using (:).
Slicing allows you to create a new tuple containing a subset of the original tuple’s elements. It uses the syntax tuple[start:end], where start is the index of the first element you want to include, and end is the index of the first element you want to exclude.
Look Example;
tuples = (1, 2, 3, 4, 5) x = tuples[1:4] print(x) # Returns (2, 3, 4)
If you don’t specify the first index, the range starts form index 0, then you can use this method that given in example below;
tuples = (1, 2, 3, 4, 5) x = tuples[:2] print(x) # Returns (1, 2)
If you don’t specify the last index, the range ends with the last item of the tuple, then you can use this method that given in example below;
tuples = (1, 2, 3, 4, 5) x = tuples[2:] print(x) # Returns (3, 4, 5)
Looping Through a Tuple
You can iterate through the elements of a tuple using a for loop. This is particularly useful when you want to perform the same operation on each item in the tuple.
Look Example;
tuples = (1, 2, 3, 4, 5) for item in tuples: print(item)
Getting the Length of a Tuple
To find the number of elements in a tuple, you can use the built-in len() function:
tuples = (1, 2, 3, 4, 5, 6, "Coding") print(len(tuples)) #Output- 7
Checking If an Item Exists in a Tuple
To check if a specific item exists in a tuple, you can use the in operator. It returns True if the item is present, otherwise False.
Look Example;
tuples = (1, 2, 3, 4, 5) if 3 in tuples: print("3 is in the tuple") else: print("3 is not in the tuple")
This code will output “3 is in the tuple” because 3 is one of the elements in the tuple.
Conclusion;
Tuples are versatile data structures in Python, offering immutability and ordered collections. They are used in various scenarios, such as representing coordinates, dates, or any other data that should not change once defined. Understanding how to create, access, and manipulate tuples is essential for Python programmers, as it enables efficient and reliable data handling in their programs. I hope you have understood this tutorial, but if you have any doubt don’t hesitate to ask.
Learn More;
0 Comments