Variable in C
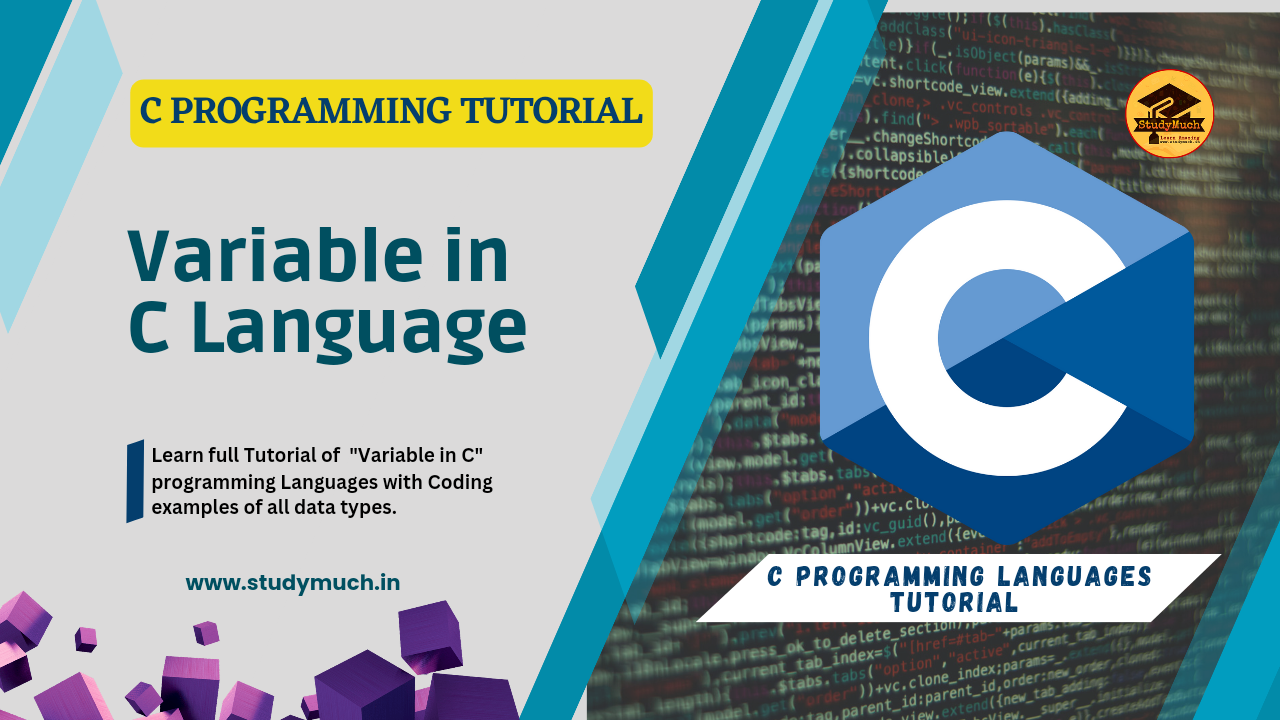
Variable in C
C is a popular programming language that is widely used in various fields such as software development, game development, and system programming. It is a high-level language that offers features like easy syntax, fast execution, and efficient memory management. In this blog post, we will discuss one of the essential concepts in C programming, such as what is variable in C, Rules to create variable, Types of Variables, and Declaring Variable in C Programming Language.
What is Variable?
A variable is a named memory location that holds a value. It is used to store data that can be modified or accessed during the program execution. The value stored in a variable can be of different types such as integers, floating-point numbers, characters, etc. In C, we declare a variable before using it. The declaration specifies the type and name of the variable.
To declare a variable in C, we use the following syntax:
data_type variable_name;
Here, data_type specifies the type of the variable, and variable_name is the name we give to the variable. For example, to declare an integer variable named x, we use the following statement:
int x;
Similarly, we can declare variables of different types like float, char, double, etc.
Rules for creating variable in C
-
A variable can have alphabets, digits, and underscore.
-
A variable name can start with the alphabet, and underscore only. It can’t start with a digit.
-
No whitespace is allowed within the variable name.
-
A variable name must not be any reserved word or keyword, e.g. int, float, etc.
Declaring Variable in C
We can declare variables of different data types like float, char, double, etc. For example, to declare a float, char, double variable named x, y and z, we use the following statement:
int x; float y; char z;
Once a variable is declared, we can assign a value to it using the assignment operator =. For example, to assign the value 10 to the variable x, value 20 to the variable y and value 30 to the variable z, we use the following statement:
int x = 10; float y = 20; char z = 30;
We can also declare multiple variables of the same type in a single statement by separating the variable names with commas. For example, to declare three integer variables named a, b, and c, we use the following statement:
int a, b, c;
Once a variable is assigned a value, we can use it in expressions. For example, we can use the variables x, y, and z in the following expressions:
int sum = x + y; float product = y * z;
It is important to note that we cannot use a variable before declaring it. Also, the value of a variable may be undefined if we access it before assigning a value to it.
Types of Variables in C
In C programming language, variables are classified based on their scope and lifetime. The following are the types of variables in C:
-
Local Variable
-
Global Variable
-
Static Variable
-
Automatic Variable
-
Register Variable
Local Variables: Local variables are declared inside a function or a block and have a local scope. They can be accessed only within the function or block in which they are declared. Local variables are created when the function or block is executed and destroyed when the function or block completes execution. They are used to hold temporary values and cannot be accessed by other functions.
Here’s an Example:
#include <stdio.h> void exampleFunction() { int localVariable = 10; printf("Local variable value: %d\n", localVariable); } int main() { exampleFunction(); return 0; }
In this example, localVariable is a local variable declared inside the function exampleFunction().
Global Variables: Global variables are declared outside any function or block and have a global scope. They can be accessed from any function or block in the program. Here’s an example:
#include <stdio.h> int globalVariable = 10; void exampleFunction() { printf("Global variable value: %d\n", globalVariable); } int main() { exampleFunction(); return 0; }
In this example, globalVariable is a global variable declared outside any function or block.
Static Variables: Static variables are declared inside a function or a block with the static keyword. They have a local scope and retain their value even after the function or block completes execution. Here’s an example:
#include <stdio.h> void exampleFunction() { static int staticVariable = 0; staticVariable++; printf("Static variable value: %d\n", staticVariable); } int main() { exampleFunction(); exampleFunction(); exampleFunction(); return 0; }
In this example, staticVariable is a static variable declared inside the function exampleFunction().
Automatic Variables: Automatic variables are declared inside a function or a block without any storage class specifier. They have a local scope and are created when the function or block is executed and destroyed when the function or block completes execution. Here’s an example:
#include <stdio.h> void exampleFunction() { int automaticVariable = 10; printf("Automatic variable value: %d\n", automaticVariable); } int main() { exampleFunction(); return 0; }
In this example, automaticVariable is an automatic variable declared inside the function exampleFunction().
Register Variables: Register variables are declared inside a function or a block with the register keyword. They have a local scope and are stored in the CPU register instead of the main memory. Here’s an example:
#include <stdio.h> void exampleFunction() { register int registerVariable = 10; printf("Register variable value: %d\n", registerVariable); } int main() { exampleFunction(); return 0; }
In this example, registerVariable is a register variable declared inside the function exampleFunction().
In conclusion, understanding the variable and different types of variables in C and their usage is crucial for writing efficient programs. So, in this tutorial you have learned Variable of C programming language, I hope you have understood this “Variable in C” Tutorial. But if you have any doubt regarding this topic, then you can ask in the comment section without any hesitation.
Learn Related;
0 Comments