C Identifiers
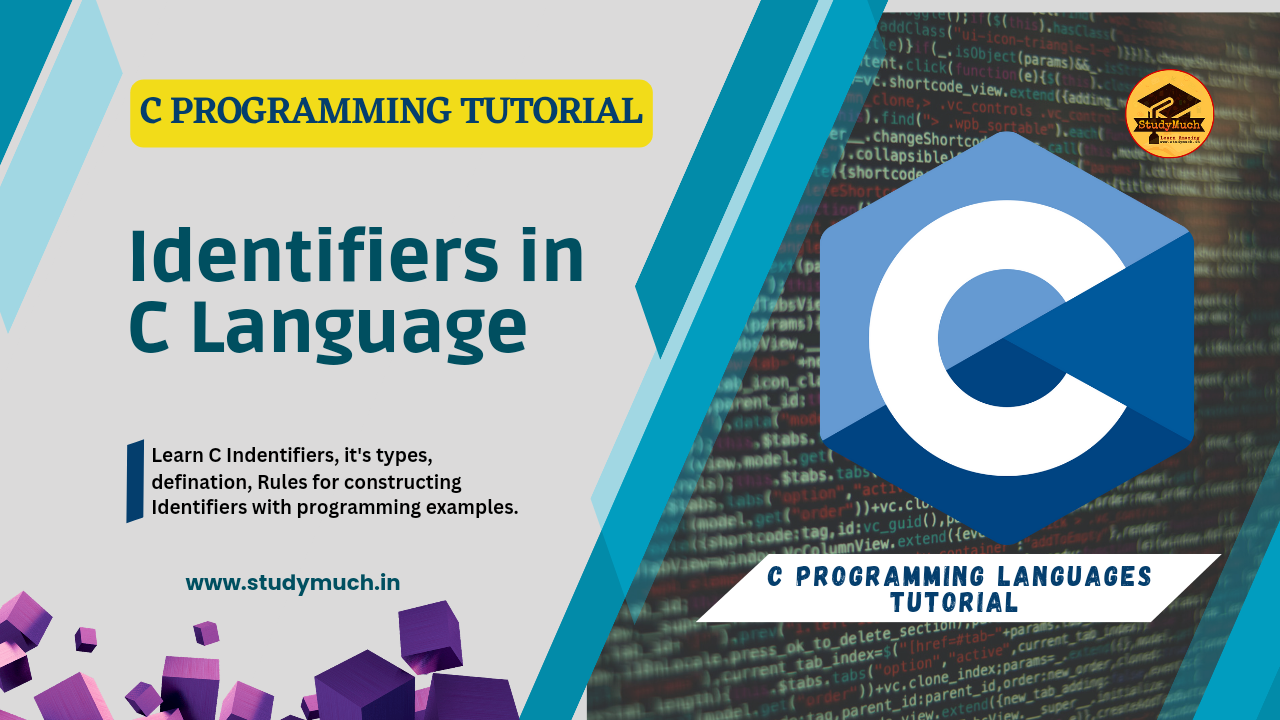
Understanding C Identifiers: Rules, Types, and Examples
Introduction:
C is a powerful and widely used programming language, follows a set of rules for constructing identifiers. Identifiers are names used to identify variables, functions, labels, and other user-defined entities in a C program. In this blog post, we will delve into the rules for constructing C identifiers, explore the different types of identifiers, and provide programming examples to illustrate their usage.
What is Identifier in C?
In C programming, an identifier is a user-defined name used to identify variables, functions, labels, and other entities in a program. It consists of letters (both uppercase and lowercase), digits, and underscores (_). The first character must be a letter or an underscore, and identifiers are case-sensitive. Identifiers provide unique and meaningful names to program elements, facilitating effective referencing and manipulation.
Rules for Constructing C Identifiers:
- Valid characters: Identifiers in C can consist of letters (both uppercase and lowercase), digits, and the underscore character (_). The first character of an identifier must be a letter or an underscore.
- Length: C identifiers can have any length, but only the first 31 characters are significant. C is a case-sensitive language, so uppercase and lowercase letters are considered distinct.
- Reserved words: Identifiers cannot be the same as reserved words, which are predefined by the C language (e.g., if, while, int). Using reserved words as identifiers will result in a compilation error.
- Meaningful names: Choose meaningful and descriptive names for identifiers to enhance code readability and maintainability. For example, a variable storing a person’s age could be named personAge.
Types of Identifiers:
1. Variable identifiers: These are used to represent memory locations that hold values during program execution. Variable identifiers follow the rules for constructing C identifiers and can be declared for various data types like int, float, char, etc. For instance:
int count; float temperature; char initial;
2. Function identifiers: Function identifiers are used to represent user-defined functions. They follow the same rules as variable identifiers. Here’s an example:
int calculateSum(int a, int b) { return a + b; }
3. Constant identifiers: Constants are fixed values that cannot be modified during program execution. Constant identifiers are typically defined using the const keyword and follow the same rules as variable identifiers. An example:
const int MAX_VALUE = 100;
4. Label identifiers: Labels are used in control statements like goto and switch to specify a target location within the program. Label identifiers follow the same rules as variable identifiers. Example:
void printNumbers() { int i; for (i = 0; i < 10; i++) { if (i == 5) { goto end; } printf("%d ", i); } end: printf("End of program"); }
Difference Between Keywords and Identifier;
Keyword |
Identifier |
Keyword is a pre-defined word. | The identifier is a user-defined word |
It must be written in a lowercase letter. | It can be written in both lowercase and uppercase letters. |
Its meaning is pre-defined in the c compiler. | Its meaning is not defined in the c compiler. |
It is a combination of alphabetical characters. | It is a combination of alphanumeric characters. |
It does not contain the underscore character. | It can contain the underscore character. |
Programming Examples:
Let’s see a practical example of using identifiers in a C program:
#include <stdio.h> int main() { int radius = 5; float area, circumference; area = 3.14 * radius * radius; circumference = 2 * 3.14 * radius; printf("Area: %f\n", area); printf("Circumference: %f\n", circumference); return 0; }
In the above example, we define variable identifiers (radius, area, circumference) to calculate the area and circumference of a circle. We then use the printf function (identified by its name) to display the calculated values.
Conclusion:
Understanding and following the rules for constructing C identifiers is crucial for writing clean and error-free code. By choosing meaningful names and using the appropriate types of identifiers, you can enhance the readability and maintainability of your C programs. Incorporating these principles into your coding practices will contribute to more efficient and professional programming.
Learn More;
0 Comments