C Operator
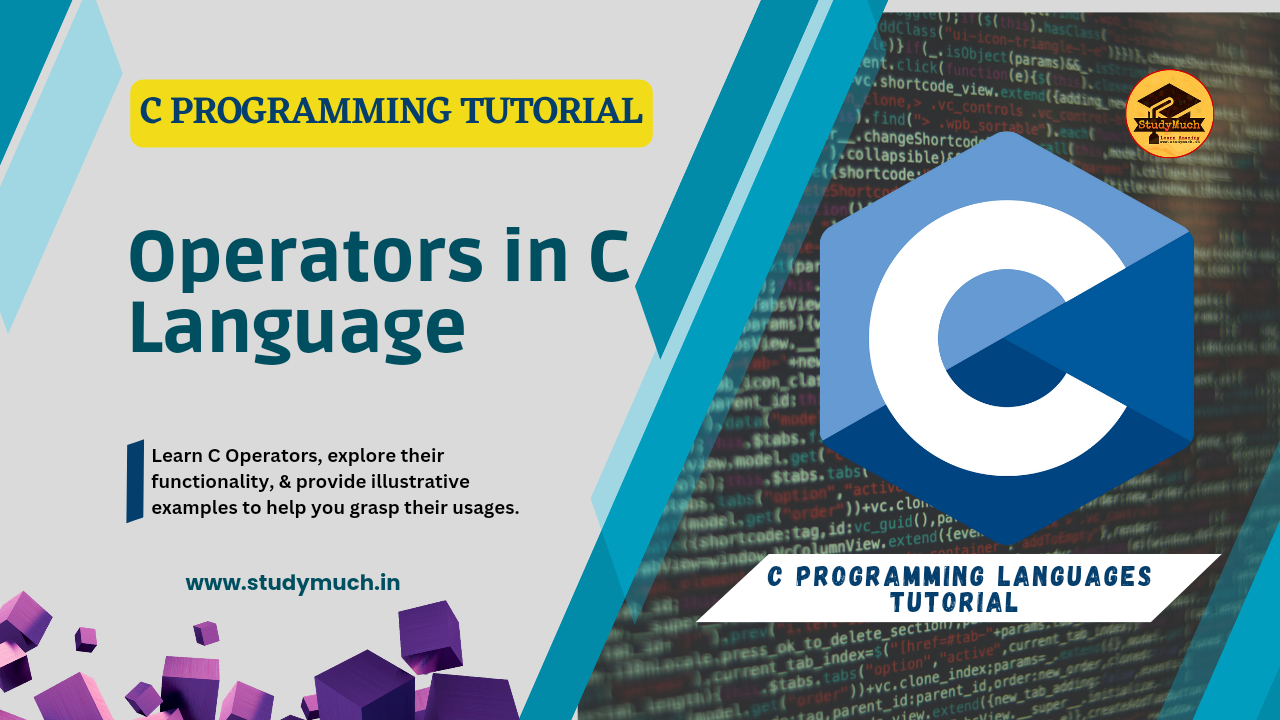
C Operator: A Comprehensive Guide with Examples
Introduction:
In the realm of the C programming language, operators play a crucial role in manipulating data and performing various operations. Among the plethora of operators available, the C operator stands out as a fundamental building block for performing arithmetic, logical, and assignment operations. In this blog post, we will learn C operators, explore their functionality, and provide illustrative examples to help you grasp their usage and power.
What is Operator?
In the C programming language, an operator is a symbol that represents a specific operation to be performed on one or more operands. Operators are used to manipulate data, perform calculations, compare values, and assign values to variables.
Arithmetic Operators:
Arithmetic operators are used to perform basic mathematical operations. In C, the common arithmetic operators are:
- Addition (+): Performs addition of two operands.
- Subtraction (-): Performs subtraction of one operand from another.
- Multiplication (*): Performs multiplication of two operands.
- Division (/): Performs division of one operand by another.
- Modulus (%): Returns the remainder after division.
Example:
#include <stdio.h> int main() { int num1, num2, sum, difference, product, quotient, remainder; printf("Enter two numbers: "); scanf("%d %d", &num1, &num2); // Arithmetic operations sum = num1 + num2; difference = num1 - num2; product = num1 * num2; quotient = num1 / num2; remainder = num1 % num2; // Displaying the results printf("Sum: %d\n", sum); printf("Difference: %d\n", difference); printf("Product: %d\n", product); printf("Quotient: %d\n", quotient); printf("Remainder: %d\n", remainder); return 0; }
Output;
Enter two numbers: 25 7 Sum: 32 Difference: 18 Product: 175 Quotient: 3 Remainder: 4
Relational Operators:
Relational operators are used to compare values and determine the relationship between them. The common relational operators in C are:
- Equal to (==): Checks if two operands are equal.
- Not equal to (!=): Checks if two operands are not equal.
- Greater than (>): Checks if the left operand is greater than the right operand.
- Less than (<): Checks if the left operand is less than the right operand.
- Greater than or equal to (>=): Checks if the left operand is greater than or equal to the right operand.
- Less than or equal to (<=): Checks if the left operand is less than or equal to the right operand.
Example:
#include <stdio.h> int main() { int num1, num2; printf("Enter two numbers: "); scanf("%d %d", &num1, &num2); // Relational operations and display the results printf("%d > %d is %d\n", num1, num2, num1 > num2); printf("%d < %d is %d\n", num1, num2, num1 < num2); printf("%d >= %d is %d\n", num1, num2, num1 >= num2); printf("%d <= %d is %d\n", num1, num2, num1 <= num2); printf("%d == %d is %d\n", num1, num2, num1 == num2); printf("%d != %d is %d\n", num1, num2, num1 != num2); return 0; }
Output;
Enter two numbers: 10 5 10 > 5 is 1 10 < 5 is 0 10 >= 5 is 1 10 <= 5 is 0 10 == 5 is 0 10 != 5 is 1
Logical Operators:
Logical operators are used to perform logical operations on boolean values. The common logical operators in C are:
- Logical AND (&&): Returns true if both operands are true.
- Logical OR (||): Returns true if at least one of the operands is true.
- Logical NOT (!): Reverses the logical state of the operand.
Example:
#include <stdio.h> int main() { int num1, num2; printf("Enter two numbers: "); scanf("%d %d", &num1, &num2); // Logical operations and display the results printf("(%d > 0) && (%d > 0) is %d\n", num1, num2, (num1 > 0) && (num2 > 0)); printf("(%d > 0) || (%d > 0) is %d\n", num1, num2, (num1 > 0) || (num2 > 0)); printf("!(%d > %d) is %d\n", num1, num2, !(num1 > num2)); return 0; }
Output;
Enter two numbers: 5 -3 (5 > 0) && (-3 > 0) is 0 (5 > 0) || (-3 > 0) is 1 !(5 > -3) is 0
Assignment Operators:
Assignment operators are used to assign values to variables. The common assignment operators in C are:
- Assignment (=): Assigns the value on the right to the variable on the left.
- Addition assignment (+=): Adds the value on the right to the variable on the left and assigns the result to the left variable.
- Subtraction assignment (-=): Subtracts the value on the right from the variable on the left and assigns the result to the left variable.
- Multiplication assignment (*=): Multiplies the variable on the left by the value on the right and assigns the result to the left variable.
- Division assignment (/=): Divides the variable on the left by the value on the right and assigns the result to the left variable.
- Modulus assignment (%=): Calculates the modulus of the variable on the left by the value on the right and assigns the result to the left variable.
Example:
#include <stdio.h> int main() { int num1, num2; printf("Enter two numbers: "); scanf("%d %d", &num1, &num2); // Assignment operations num1 += num2; // num1 = num1 + num2 printf("num1 after addition: %d\n", num1); num1 -= num2; // num1 = num1 - num2 printf("num1 after subtraction: %d\n", num1); num1 *= num2; // num1 = num1 * num2 printf("num1 after multiplication: %d\n", num1); num1 /= num2; // num1 = num1 / num2 printf("num1 after division: %d\n", num1); num1 %= num2; // num1 = num1 % num2 printf("num1 after modulus: %d\n", num1); return 0; }
Output;
Enter two numbers: 10 3 num1 after addition: 13 num1 after subtraction: 10 num1 after multiplication: 30 num1 after division: 10 num1 after modulus: 1
Conclusion:
Understanding and mastering the C operator is essential for performing various operations in the C programming language. By leveraging arithmetic, relational, logical, and assignment operators, you can manipulate data, compare values, and assign values to variables effectively. Through the examples provided in this blog post, you now have a solid foundation to apply these operators in your own C programs.
Remember to consider operator precedence and use parentheses when necessary to control the order of operations. Continually practice and explore different scenarios to enhance your understanding of C operators. With time and experience, you’ll become proficient in utilizing these powerful tools to develop robust and efficient C programs.
Learn More;
1 Comment
tlovertonet · May 6, 2024 at 5:07 am
Good day very nice web site!! Man .. Beautiful .. Amazing .. I’ll bookmark your web site and take the feeds also…I’m glad to search out a lot of helpful information right here in the submit, we’d like work out more strategies on this regard, thank you for sharing.