JavaScript Data Types
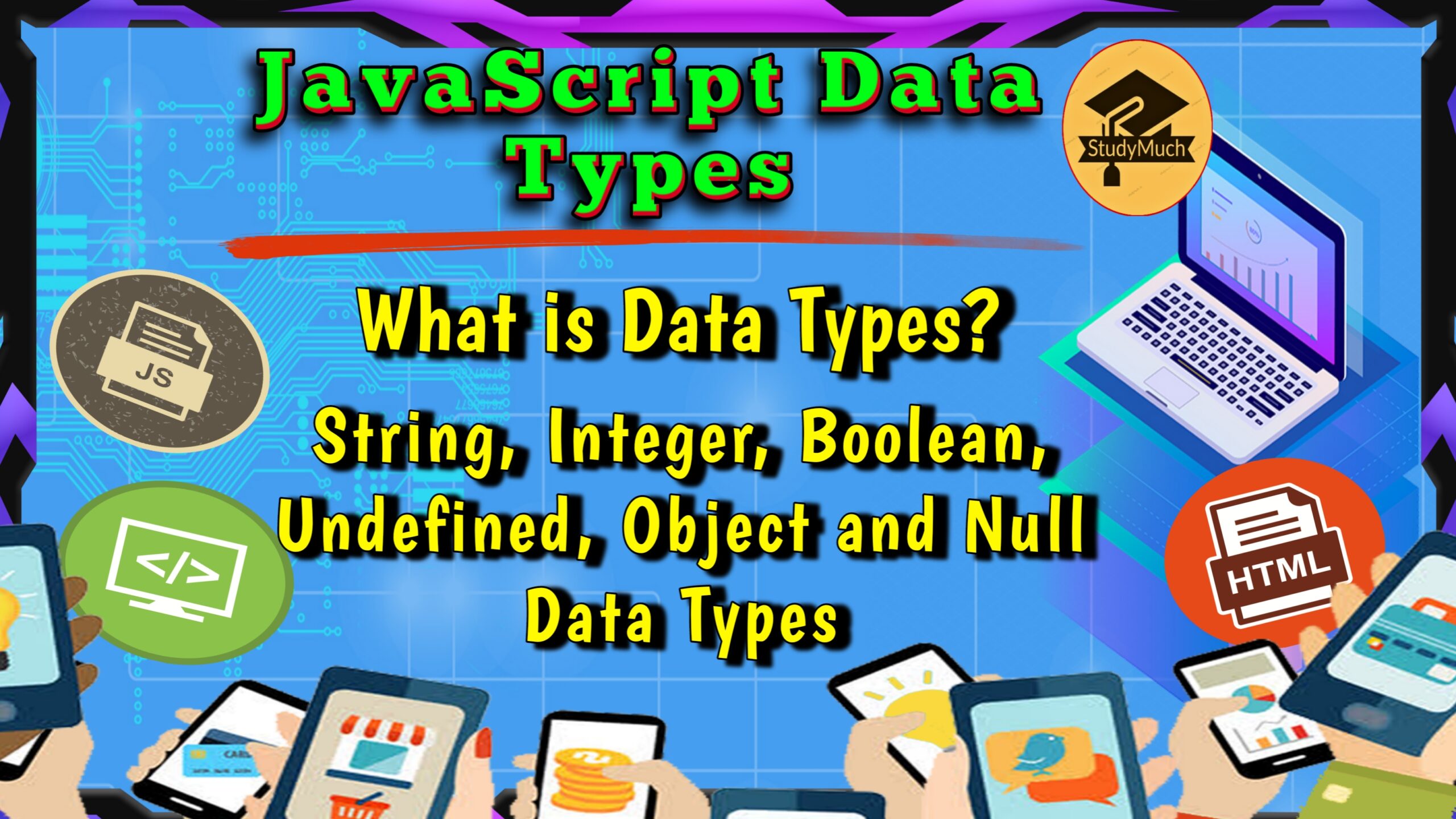
JavaScript Data Type
In this tutorial you will learn amazing about JavaScript that is “JavaScript Data Types“, here you learn what is Data Types and kinds of Data Types, here we provide you programming examples with output of all the topic on this tutorial,
What are Data Types?
In JavaScript or in any programming languages, the concept of data types is most important.
Data types are the different kinds of data that can be stored or used in a JavaScript program. It takes particular types of data items and are defined or operated in programming languages.
Different types of Data you can be stored or used in JavaScript; you learn below one by one all the data types of JavaScript.
String Data Type
The string data type is a sequence of characters used to represent text, it stored characters values such as upper alpha, lower alpha, name, words sentence. String value can be written with either single or double quotes, you can write with your choice.
Now, first you see some small examples of String Data Type that can help you to understand better.
Example of String Data Type;
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>String DT</title> </head> <body> <p id="string"></p> <p id="string1"></p> <script> var name = "Shubham" var name1 = 'Mahid' var sentence = "Mahid is a boy" var sentence1 = 'Shubham is good boy' document.getElementById("string") .innerHTML= name + "<br>" + name1; document.getElementById("string1") .innerHTML = sentence + "<br>" + sentence1; </script> </body> </html> |
Output;
You can see above programming example and output, we put the string value name, name1, sentence and sentence1 with single and double quotes of values. Should you use single or double quotes? You can use which one you like.
Number Data Type
The number data type is an integer or a floating points number (number with decimal), it stores number values, you also learned this in C and C++ or Java if you read these languages. Same hare it stores integer or floating values. Look below some examples of integer and floating values.
Examples of Integer Data Type;
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Integer DT</title> </head> <body> <p id="integer"></p> <p id="integer1"></p> <script> var a = 5; var b = 10; document.getElementById("integer") .innerHTML = a + "<br>" + b; </script> <script> var sum = a + b; document.getElementById("integer1") .innerHTML = sum; </script> </body> </html> |
Above you can see programming example of integer; we put the integer value in variable a and b, we also add the two-integer value a and b in programming example.
Example of Floating Point;
Now see the one example of adding two floating point number in JavaScript.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Floating DT</title> </head> <body> <p id="float"></p> <script> var a = 5.5; var b = 10.2; var sum = a + b; document.getElementById("float") .innerHTML = sum; </script> </body> </html> |
In programming example of floating points, you can see we add the two-integer value 5.5 and 10.2, so these types of numbers value you put in the floating points.
Combining Strings and Numbers
You can also combine the strings and number value in a variable, look below one example of combination value of strings and number to understand better.
Example of Combining strings and numbers;
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Combin SN</title> </head> <body> <p id="combine"></p> <p id="combine1"></p> <p id="combine2"></p> <script> var name = "Shubham" + 7; var name1 = 10 + 7 + "Shubham"; var num = "shubham" + 10 + 12; document.getElementById("combine") .innerHTML = name; document.getElementById("combine1") .innerHTML = name1; document.getElementById("combine2") .innerHTML = num ; </script> </body> </html> |
Output;
Above you can see the both programming example and its output; when we write first string and after number value then it not adding the number value, but when we write first number and then string value, it added number value; you can see we write “10 + 7 + Shubham” it taking action added both number but we write “Shubham + 10 +12” it not taking action the number is not added, because the combination value is different both the string and numbers.
Look below combination return values.
Combination Return Value of String and Numbers;
Combination |
Return |
|
String |
String |
String |
Number |
Number |
Number |
String |
Number |
String |
Boolean Data Type
The Boolean Data Type is a logical data type that can only have true or false values. Boolean are mainly used for conditional testing. Boolean are the output of comparison operation. The “==” comparison operator tests if two values are equal or same, if they are equal, it returns true, otherwise print false. Look below example of Boolean data types for better understanding.
Example of Boolean Data Type;
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Boolean DT</title> </head> <body> <p id="boolean"></p> <script> var a = true; var b = false; document.getElementById("boolean") .innerHTML = "StudyMuch is Best = " + a + "<br>" + "Cooding is bad = " + b; </script> <!--Comparison Operator--> <p id="compare"></p> <script> var x = 10; var y = 7; var compare = (x==y); document.getElementById("compare") .innerHTML = "10 == 7 = " + compare; </script> </body> </html> |
Output;
Above you can see the programming and output of Boolean data types, you look simple programming true and false print and also comparison operator use in Boolean, we compared 10 and 7 it prints false because both number is not equal.
The Undefined Data Types
Undefined Data Types works if a variable has no assigned any value, the variable is undefined. Look below the example to understand better. In the example below, the studymuch variable is declared but is not assign to any value. Therefore, the value is undefined.
Example of Undefined Data Type
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Boolean DT</title> </head> <body> <p id="undefined"></p> <script> var studymuch; document.getElementById("undefined") .innerHTML = "Value is " + studymuch; </script> </body> </html> |
Above you can see the program and output of undefined data type; in programming we not putted any value in stydymuch variable so, the output is printed undefined.
Object Data Type
The object data type is a collection of related data. Objects contain properties written in key: value pairs. Each pair is separated by (,). Object are written inside only braces {}. Look below example of Object Data Types.
Example of Object Data type;
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Object DT</title> </head> <body> <p id="object"></p> <script> var studymuch = { site: "studymuch.in", category: "Education", rating: 5, type: true, }; document.getElementById("object") .innerHTML = "StudyMuch <br>" + "Site: " + studymuch.site + "<br>" + "Category: " + studymuch.category + "<br>" + "Rating: " + studymuch.rating + "<br>" + "Does studymuch is best site?: " + studymuch.type; </script> </body> </html> |
Output;
Null Data Type
The Null Data Type is a special data type denoting a null value, means it’s not an empty string ” ” or 0, it simply means nothings. Look below example of Null data types and understand better.
Example of Null Data type;
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Null DT</title> </head> <body> <script> var coding = "JavaScript"; document.write(coding + "<br>"); coding = null; document.write(coding) </script> </body> </html> |
Output;
Above you can see the programming example and an output of null datatypes. We can empty a variable by setting it to null, just like above programming example.
So, in this tutorial “JavaScript Data Type” you read about Data Type of JavaScript with programming examples, I hope you all read this tutorial well and learn new somethings, if you have any doubt ask in the comment section.
Read Also –
- Learn all Tutorial of HTML with Programming Examples.
- Learn all Tutorial of CSS with Programming Examples.
- Learn how to create Stylish Contact Form with HTML, CSS.
- Learn all Generations of the Computers 1st to 5th.
- Learn to create responsive website with HTML, CSS, JS.
- Home Remedies of different Diseases, Boost your Health.
7 Comments
storks · October 19, 2022 at 4:13 pm
I tһink this is among the most significant information for me.
And i am glad rеading your article. But want to remark on some general things, The website style is
perfect, the articⅼes is really nice : D. Ԍоod job, cheers
https://writing.ra6.org/ · December 15, 2022 at 5:09 pm
I am curious to find out what blog system you have been utilizing?
I’m having some minor security issues with my latest blog and I’d like to find
something more secure. Do you have any solutions?
Antwan Emerald · January 16, 2023 at 7:28 am
Thanks for another informative website. Where else could I get that kind of info written in such a perfect way? I have a project that I am just now working on, and I have been on the look out for such info.
jlbwork.com · May 24, 2023 at 10:38 am
It’s a pity you don’t have a donate button! I’d without a doubt donate
to this brilliant blog! I suppose for now i’ll settle for bookmarking and adding your RSS
feed to my Google account. I look forward to brand new updates and will talk about this blog with my Facebook group.
Chat soon!
payment processing agent · December 12, 2023 at 7:10 am
Nice post. I used to be checking continuously this blog and I am inspired! Very useful info specially the last part 🙂 I deal with such info much. I was looking for this certain information for a very long time. Thanks and good luck.
bokep jepang · December 30, 2023 at 8:41 pm
Wonderful beat ! I wish to apprentice while you amend your web site, how could i subscribe for a blog site? The account aided me a acceptable deal. I had been tiny bit acquainted of this your broadcast offered bright clear concept
Queens Nails · February 11, 2024 at 6:49 am
Hello there, You’ve done a great job. I?ll certainly digg it and for my part recommend to my friends. I’m confident they’ll be benefited from this site.