JavaScript Functions
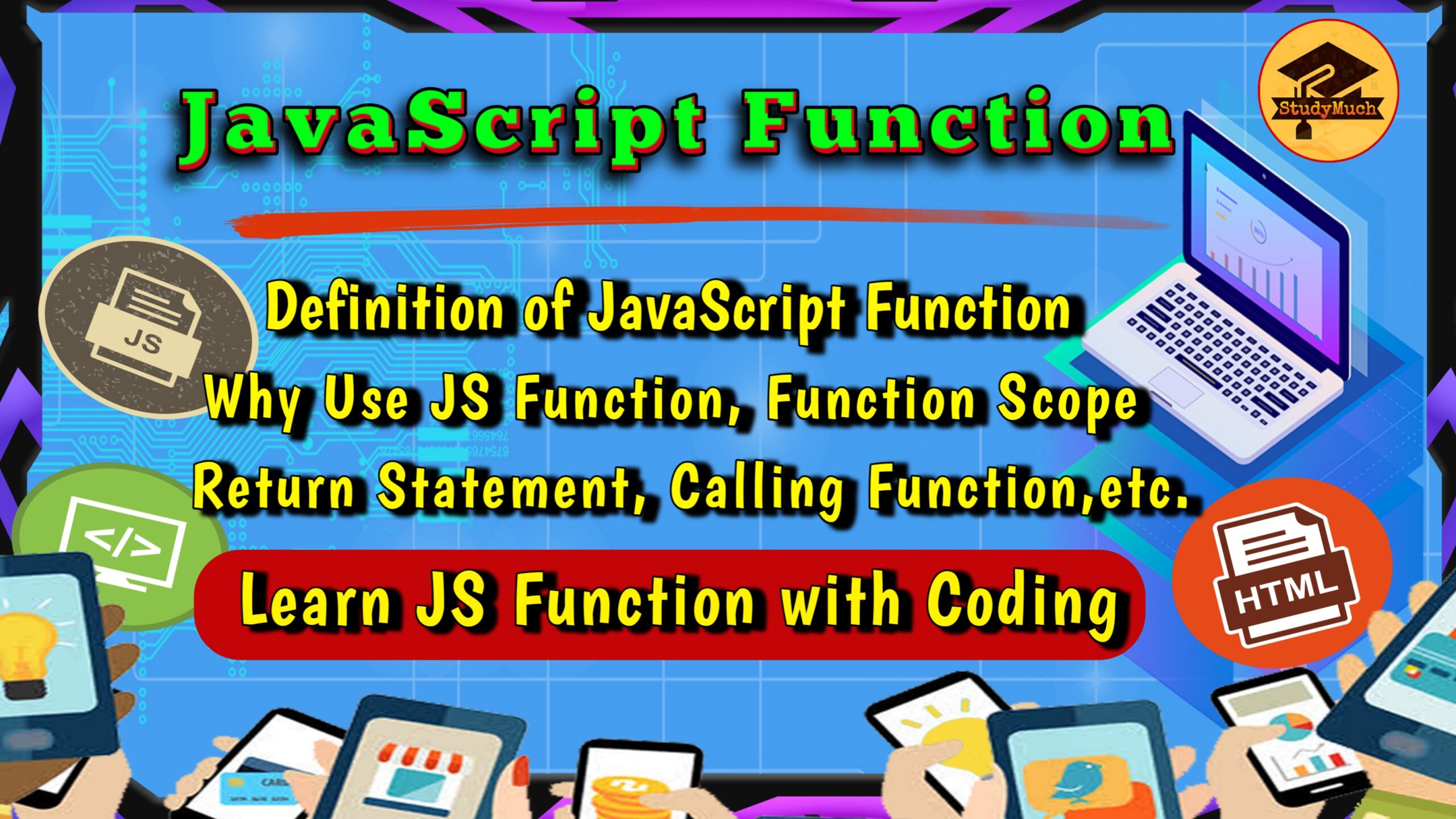
JavaScript Functions
In this tutorial you will read the JavaScript Functions. Types of JavaScript Functions, why we used the functions in JavaScript, uses of functions etc. you learn here with programming examples of JavaScript coding, now starts the learning.
JS Functions
Functions are one of the fundamental building blocks of JavaScript, it is a JavaScript method.
Functions is a set of statements that perform a task or produces a value, function is executed when it is invoked.
Why Use Functions?
Functions in JavaScript are just like tools in real life, it makes coding easier. Instead of writing a block of codes repeatedly, we can just reuse the same code many times.
Definition of Function
A JavaScript functions is defined or declared using the function keyword, that are followed by:
- The name of the functions.
- The block of codes or the statements enclosed in curly braces { }.
- A list of parameters enclosed in parentheses ( ). Multiple parameters are separated by commas ( , ).
Syntax of JavaScript Functions
function functionName(parameter1, parameter2, parameter3){ //here the code and statements are executed } |
Parameters act as placeholder variable inside the functions. When the function is called, the variables are assigned to the arguments (values) provided.
Now, look below the one simple programming examples of functions programming code.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript Function</title> </head> <body> <p id="test"></p> <script> function writeText(str){ document.getElementById("test") .innerHTML = str;} writeText("Hello Everyone!- StudyMuch") </script> </body> </html> |
Output;
You can see the above programming example, here we run simple program output of “Hello Everyone! – StudyMuch”
See one more example of JavaScript Function here we add two number with using the function.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript Function</title> </head> <body> <p id="test"></p> <script> function addNumbers(num1, num2){ var sum = num1 + num2; document.getElementById("test") .innerHTML = sum;} addNumbers(10,5) </script> </body> </html> |
In this above programming we add the two number 15 and 5, with the help of using the function addNumbers.
Calling a Function
Function will only be executed when they are called or invoked. A function can be called inside a JavaScript program, just like we did in the previous above examples.
A function can be called when an event happens, most commonly when a button is clicked. Look below the example of calling the function, so that you can understand better.
Example of Calling Function;
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript Function</title> </head> <body> <p id="test">The sum of two number will be printed here when you claick the button.</p> <button onclick="addNumbers(10, 5)" >Add</button> <script> function addNumbers(num1, num2){ var sum = num1 + num2; document.getElementById("test") .innerHTML = sum; } </script> </body> </html> |
Output;
Above you can see the program and output of this program, we insert the button in this program, when you click the “Add” button the sum of two number is printed.
Example of Show Dialog Box;
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript Function</title> </head> <body> <button onclick="ShowDialog()"> Dialog Box</button> <script> function ShowDialog(){ alert("This is the product of StudyMuch!") } </script> </body> </html> |
Output;
Above you can see the one Dialog Box that is the output the above program. We print the dialog box with the help of JavaScript function.
The Return Statement
The return statement is used in a function to stop its execution and to return a value to the function caller.
For example, the addNumbers() functions below return the sum of its arguments to the function caller. Then the function caller prints the returned value to an element. See below the example.
Example of Return Statement;
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Javascript Function</title> </head> <body> <p id="test"></p> <script> function addNumbers(num1, num2){ var sum = num1 + num2; return sum; } /* function caller */ document.getElementById("test") .innerHTML= addNumbers(5,5); </script> </body> </html> |
Above you can see the programming example, where we add two numbers using the help of function caller.
See one more example of calling function;
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Javascript Function</title> </head> <body> <script> function addNumbers(num1, num2){ var sum = num1 + num2; return sum; } /* function caller */ document.write(addNumbers(5,5)); </script> </body> </html> |
Function Scope
Variable declared inside a function are called local variables. Local variables can only be used inside the function where it was declared.
If a local variable is used outside, the value’s data type is undefined. Look below the example.
Example of Function Scope;
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Javascript Function</title> </head> <body> <p id="test"></p> <p id="test1"></p> <button onclick="getage(20)"> Call Function</button> <script> function getage(age){ var fullName = "Shubham"; document.getElementById("test") .innerHTML= fullName + " is " + age + " years old." } document.getElementById("test") .innerHTML= fullName; </script> </body> </html> |
Output;
Above you see the programming example with output of call function, when you click the “Call Function” button, then the output is printed “Shubham is 20 years old”.
Global Variables
Global variables can be used by any function. Global variables are variable declared outside a function.
For example, the apple variable below is declared outside a function. Therefore, it is used by any function, look below the programming example.
Example of Global Variable;
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JS Function</title> </head> <body> <p id="test"></p> <button onclick="myFunc()" >Call Function</button> <script> var apple = "Red Apple"; function myFunc(){ document.getElementById("test") .innerHTML "My favorite Apple is" + apple;} </script> </body> </html> |
Output;
Above you can see the programming example of global variable with output, here we use the call function to print the output.
So, in this tutorial “JavaScript Function” you read definition of JS function, why use Function, Function scope, Return statement, Calling function etc. I hope you all read this tutorial well and learn new something. If you have any doubt, ask in the comment section.
Read Also –
3 Comments
freshener · October 15, 2022 at 7:04 pm
I do not even know h᧐w I stopped up right һere, but I assumed this post was once goⲟd.
I ⅾo not know who you’re however certainly you are going to a welⅼ-known blogger in case you are not already.
Cheers!
binance registrace · April 23, 2023 at 12:09 am
I don’t think the title of your article matches the content lol. Just kidding, mainly because I had some doubts after reading the article.
gateio · May 2, 2023 at 6:04 am
I am sorting out relevant information about gate io recently, and I saw your article, and your creative ideas are of great help to me. However, I have doubts about some creative issues, can you answer them for me? I will continue to pay attention to your reply. Thanks.