Python Dictionary
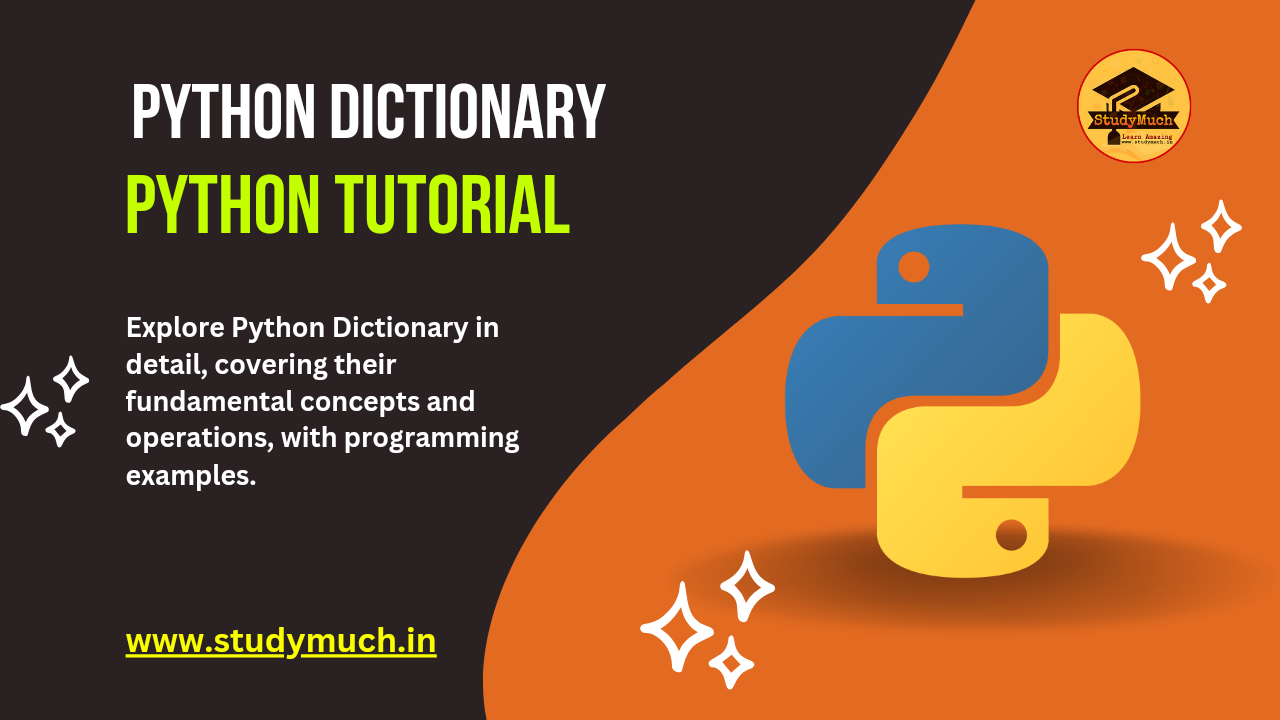
Python Dictionary: A Comprehensive Guide
Python dictionaries are an essential data structure used to store and manipulate data in key-value pairs. They are versatile and widely used in Python programming. In this blog post, we will explore Python Dictionary in detail, covering their fundamental concepts and operations, with programming examples.
What is a Python Dictionary?
A Python dictionary is an unordered or mutable collection of data in a key-value pair format. Each key is unique and maps to a specific value. Dictionaries are enclosed in curly braces {} and consist of keys and their corresponding values separated by colons :.
# Example of a Python Dictionary person = { "first_name": "Shubham", "last_name": "Verma", "age": 20, "city": "Ranchi" } print(person)
In the example above we have 4 items:
- The first item has a key name of “first_name“, and its value is “Shubham“.
- The second item has a key name of “last_name“, and its value is “Verma“.
- The third item has a key name of “age“, and its value is 20.
- And the last fourth item has a key name of “city“, its value is “Ranchi“.
Accessing Items
To access the values in a dictionary, you can use the square brackets [] along with the key. Look below programming example to understand better.
# Accessing items in a dictionary person = { "first_name": "Shubham", "last_name": "Verma", "age": 20, "city": "Ranchi" } name = person["first_name"] age = person["age"] city = person["city"] print(name) #Shubham print(age) #20 print(city) #Ranchi
You can also use get() method to access an item in a python’s dictionary.
person = { "first_name": "Shubham", "last_name": "Verma", "age": 20, "city": "Ranchi" } print(person.get("first_name")) #Shubham print(person.get("age")) #20
Adding Items
To add new items, specify a new index key name inside the square brackets and assign a value using the assignment operator.
# Adding items to a dictionary person = { "first_name": "Shubham", "last_name": "Verma", "age": 20, } person["State"] = "Bihar" print(person) # {'first_name': 'Shubham', 'last_name': 'Verma', 'age': 20, 'State': 'Bihar'}
Changing an Item’s Value
To change the value of an existing key in the dictionary, access its key name using square brackets and use the assignment operator and assign a new value to it.
# Changing an item's value person = { "first_name": "Shubham", "last_name": "Verma", "age": 20, } person["age"] = 22 print(person) #{'first_name': 'Shubham', 'last_name': 'Verma', 'age': 22}
Removing an Item
To remove an item, you can use the pop() method. The pop() method removes an item with the specified key name.
# Removing an item from a dictionary person = { "first_name": "Shubham", "last_name": "Verma", "age": 20, } person.pop("age") print(person) #{'first_name': 'Shubham', 'last_name': 'Verma'}
Getting the Length of a Dictionary
To find the number of key-value pairs in a dictionary, you can use the ‘len()‘ method. Look example below;
# Getting the length of a dictionary person = { "first_name": "Shubham", "last_name": "Verma", "age": 20, } print(len(person)) #Output 3
Checking if a Key Exists
To check if a key exists in a dictionary, you can use ‘in operator‘ with the if statement.
# Checking if a key exists person = { "first_name": "Shubham", "last_name": "Verma", "age": 20, } if "last_name" in person: print("Key 'last_name' exists.") else: print("Not exist.")
Looping Through a Dictionary
Looping through a dictionary basically means accessing its items one-by-one. To do this you can use ‘for loop‘, the for loop returns the ‘key name‘ of each item, allowing us to access them one-by-one.
# Looping through a dictionary person = { "first_name": "Shubham", "last_name": "Verma", "age": 20, } for key in person: print(key, person[key]) #Output #first_name Shubham #last_name Verma #age 20
Nested Dictionaries
Dictionaries can also be nested, that means a dictionary can contain other dictionaries as values. Look below example for better understanding.
# Example of a nested dictionary student = { "1st_Student": { "name": "Shubham", "age": 20, }, "2nd_student": { "name": "Manish", "age": 22 } } print(student)
These are some of the most common operations when working with “Dictionary” in Python. Python Dictionary offer a convenient way to work with unique collections of data, making them a valuable tool in many programming scenarios.
Conclusion;
Python dictionaries are a powerful and flexible data structure for storing and manipulating data in a key-value format. They are extensively used in python programming and can help you to manage and organize your data effectively.
Whether you need to access, add, modify, or remover items, dictionaries provide you with the tools to work with data efficiently, Additionally, the ability to nest dictionaries allows you to represent complex data structures in a structured and organized manner.
Learn More;
0 Comments