Python Functions
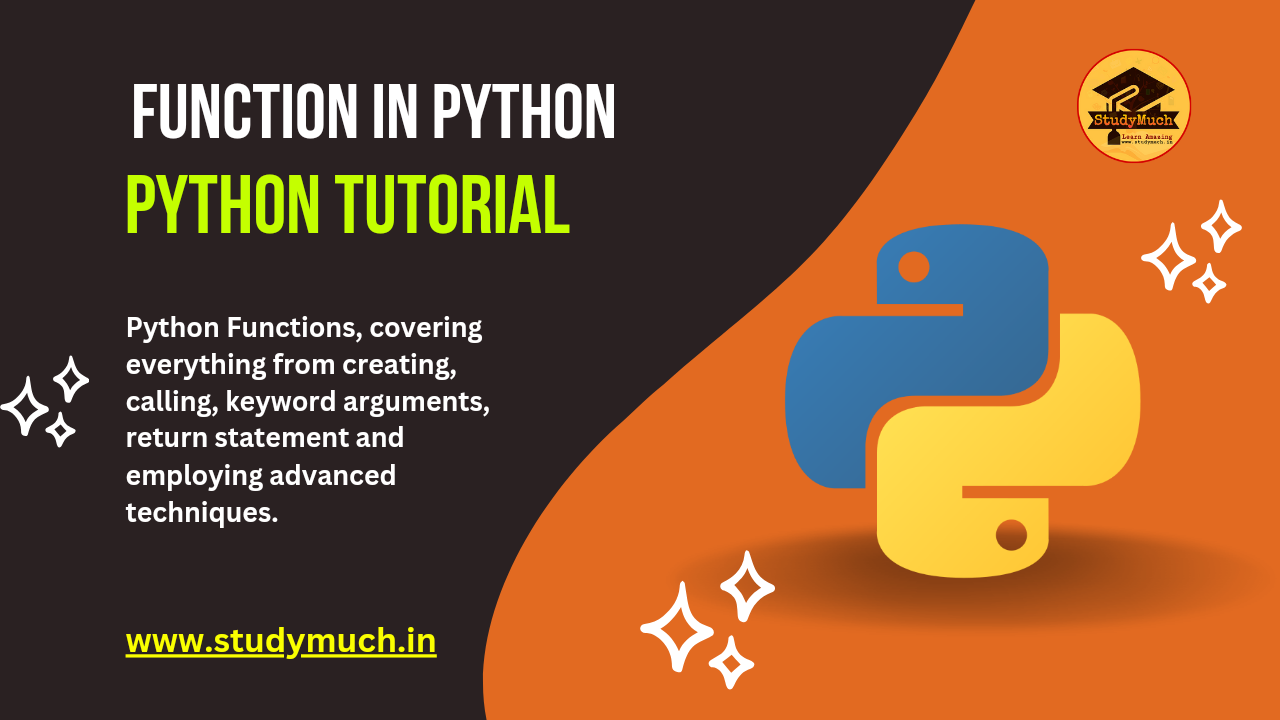
Python Functions
Python Functions are the fundamental building blocks of any robust and scalable program. They allow you to organize your code into manageable and reusable pieces, promoting modularity and readability. In this guide, we’ll delve deeper into the intricacies of Python Functions, covering everything from creating and calling functions to handling parameters, keyword arguments, return statement and employing advanced techniques.
What is Function?
A function in Python is a block of code that performs a specific task. Functions help you break your program into smaller, more manageable parts. This can make your code easier to read, understand, and maintain.
Creating a Function:
In Python, creating a function is straightforward. Use the def keyword after the function name and parentheses. The colon indicates the beginning of the function block.
To Create a function, we need the following.
- Use the def keyword.
- Write a function name.
- Round brackets (small bracket) ‘()‘ and semicolon ‘;‘.
- A function body – a group of statements.
Example of creating Function;
def my_func(): x = "Good Evening" print(x) my_func()
NOTE: A group of statement must have the same indentation level, in the example above we used 4 whitespaces to indent the function body.
Calling a Function;
To execute a function, it needs to be called, to call a function simply write its name followed by parentheses ().
Example;
def greet(): print("Hello, World!") # Call the function greet()
Function Parameters/Arguments:
Functions often require input value, known as parameters. Parameters are defined within the parentheses during function creation. When calling a function, we can pass data using parameters/arguments. A parameter is a variable declared in the function.
Example:
In this example, the hello() function has one parameter, this parameter is ‘name‘.
def hello(name): x = "Hello" + name print(x) hello("Ram")
In this above example, we are calling the hello() function with one argument, “Ram“.
Multiple Parameters/Arguments:
To use multiple parameters, separate them using commas. The arguments when calling the function should also be separated by commas.
Example;
def add_num(a, b): sum = a + b print(sum) add_num(5, 10)
In above example we passed two arguments 5 and 10.
NOTE: You will get an error if you don’t pass enough required arguments.
Default Arguments:
A function can have default arguments, it can be done using the assignment operator (=). If you don’t pass the argument, the default argument will be used instead. You can set default values for parameters, making them optional during function calls.
Example;
def hello(name = "Shubh"): x = "Hello" + name print(x) #call function with an argument hello("Manish") #Output: Hello Manish #call without an arguments (Default argument) hello() #Output: Hello Shubh
In above example we run two argument one is default argument.
Keyword Arguments:
As you may notice, the arguments passed are assigned according to their position. When using keyword arguments, the position does not matter, specify arguments by parameter name, allowing you to pass them in any order.
Example;
def func(hobby1, hobby2, hobby3): print("I Love", hobby1) print("I Love", hobby2) print("I Love", hobby3) func(hobby1="Coding", hobby2="Playing", hobby3="Reading")
Output:
I Love Coding
I Love Playing
I Love Reading
The return Statement:
The return statement is used to return a value to the function caller. Look below one example to better understanding.
#return statement def add(n1, n2): sum = n1 + n2 return sum #return print(add(10, 7)) #Output: 17
NOTE: The return statement stops the execution of a function.
def add(n1, n2): sum = n1 + n2 return sum print("This will not be printed") print(add(10, 7))
In this above code, after the return statement the “print()” function will not be printed because return statement stop the execution.
In Conclusion;
Python functions is crucial for writing clean, modular code. Whether you’re a beginner or an experienced developer, understanding function creation, parameters, default arguments, keyword arguments, and the return statement is fundamental. Use these concepts to increase the efficiency and readability of your Python code. Happy coding!
Learn More;
0 Comments