QR Code Generator with Python
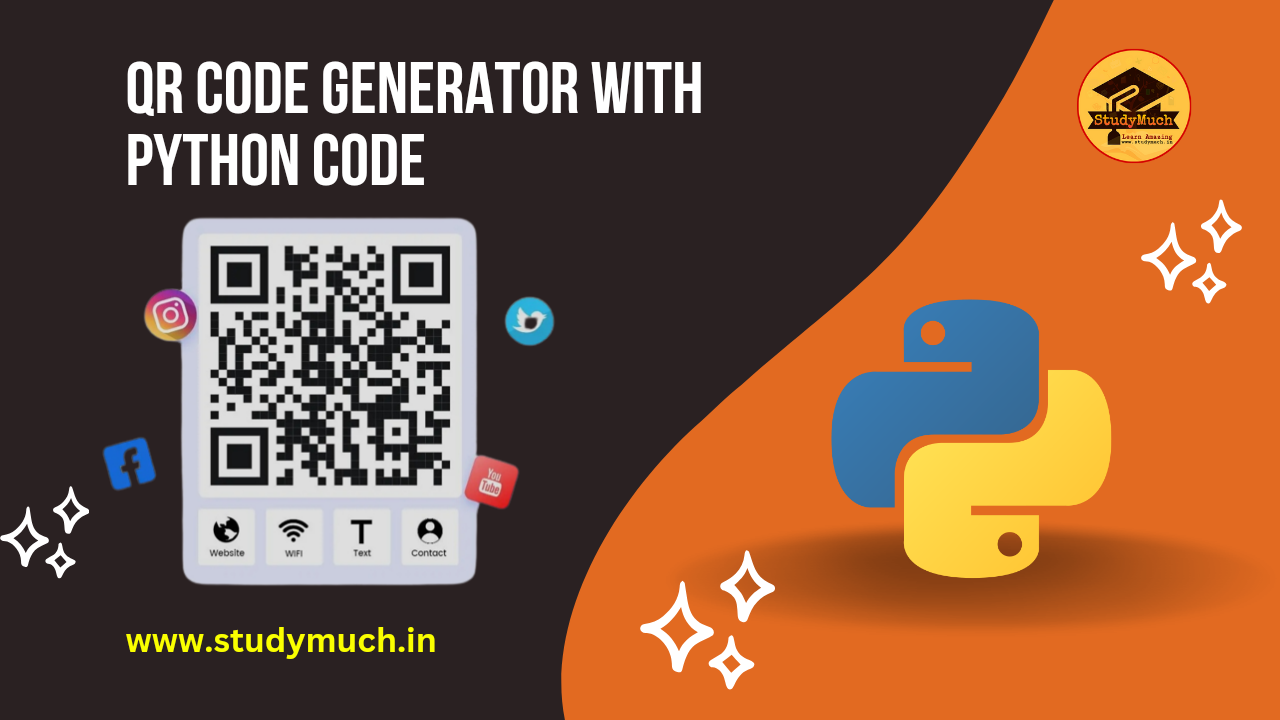
Creating a QR Code Generator with Python
QR codes, short for Quick Response codes, are two-dimensional barcodes that can store a variety of data, such as URLs, text, contact information, and more. In this tutorial, we will explore how to create a simple QR Code Generator with Python. We will use the qrcode library to generate QR codes and the tkinter library for the graphical user interface. Additionally, we will use the PIL (Pillow) library to handle image processing.
Prerequisites
Before we get started, make sure you have the following installed on your system:
- Python: You’ll need Python installed on your system. You can download it from the official Python website.
- Required Libraries: Install the necessary libraries using pip:
pip install qrcode[pil] tkinter
The Source Code
Here’s the complete source code for our QR code generator:
import qrcode import tkinter as tk from PIL import Image, ImageTk # Function to generate a QR code def generate_qr_code(): text = entry.get() # Get text from the input field qr = qrcode.QRCode(version=1, error_correction=qrcode.constants.ERROR_CORRECT_L, box_size=10, border=7) qr.add_data(text) qr.make(fit=True) img = qr.make_image(fill_color="black", back_color="white") img.save("generated_qr.png") # Save the QR code as an image # Display the QR code image above the input field qr_image = Image.open("generated_qr.png") qr_image = ImageTk.PhotoImage(qr_image) qr_label.config(image=qr_image) qr_label.image = qr_image # Create the main window window = tk.Tk() window.title("QR Code Generator -'StudyMuch'") # Create an input field for the user to enter text entry = tk.Entry(window, width=40) entry.pack(pady=10) # Create a button to generate the QR code generate_button = tk.Button(window, text="Generate QR Code", command=generate_qr_code) generate_button.pack() # Create a label to display the QR code image qr_label = tk.Label(window) qr_label.pack() # Start the main loop window.mainloop()
Code Description;
Let’s break down the code step by step for your better understanding.
1. Importing Libraries
We begin by importing the required libraries. qrcode is used for generating QR codes, tkinter is for creating the graphical user interface, and PIL (Pillow) helps us handle image processing.
2. generate_qr_code Function
This function is responsible for generating the QR code. It does the following:
- Retrieves the text entered by the user in the input field.
- Creates a QR code object with specified properties.
- Generates the QR code image with black squares on a white background.
- Saves the QR code as an image named “generated_qr.png.”
- Displays the generated QR code image above the input field using tkinter.
3. Creating the Main Window
We create the main application window using tkinter and set its title to “QR Code Generator.”
4. Input Field
We create an input field (tk.Entry) where the user can enter the text they want to encode in the QR code.
5. Generate Button
A button (tk.Button) labeled “Generate QR Code” triggers the generate_qr_code function when clicked.
6. QR Code Image Label
A label (tk.Label) is used to display the generated QR code image.
7. Main Loop
We start the main loop with window.mainloop(), which allows the user to interact with the application.
Running the Application
Save the code to a Python file (for example, qr_code_generator.py) and run it using your Python interpreter. You will see a window where you can input text and generate the QR code.
That’s it! You now have a simple QR code generator built with Python. You can further improve this application by customizing the user interface and adding more features as needed. I hope you enjoyed creating QR code with our given codes.
Learn More;
0 Comments